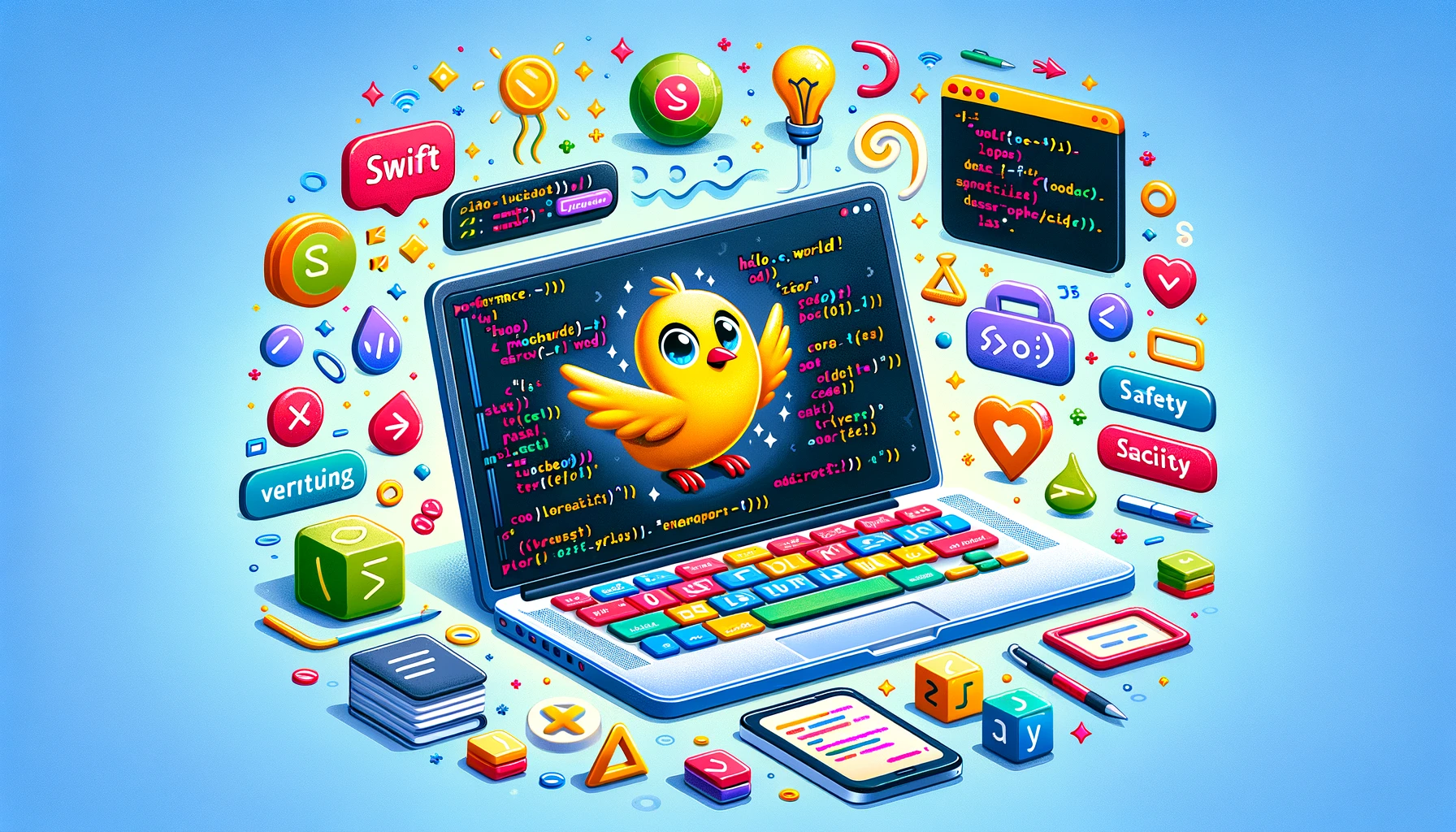
Swift, Apple’s programming language, is designed to be powerful yet easy to learn. In this guide, we’ll explore what makes Swift unique, cover the basics, and walk through some practical examples. By the end, you’ll have a solid understanding of why Swift is a go-to language for iOS development.
What is Swift?
Swift is a programming language created by Apple in 2014. It’s used primarily for developing iOS, macOS, watchOS, and tvOS applications. Swift is known for being fast, safe, and expressive. It’s designed to be beginner-friendly, making it a great choice for new programmers.
Why Choose Swift?
- Easy to Learn: Swift’s syntax is simple and clean, making it accessible for beginners.
- Performance: Swift is designed to be fast. It performs better than many other programming languages.
- Safety: Swift helps developers avoid common coding errors, making your apps more reliable.
- Interoperability: Swift works well with Objective-C, the older language used for Apple development.
Guide to R Programming Language you need to know.
Getting Started with Swift
To start programming in Swift, you need Xcode, Apple’s development environment. Xcode provides all the tools necessary to build apps for Apple devices. You can download Xcode from the Mac App Store.
Hello, World!
Let’s start with a simple “Hello, World!” program to get a feel for Swift’s syntax.
import UIKit
print("Hello, World!")
In this example, import UIKit
brings in essential libraries, and print("Hello, World!")
outputs the text to the console.
Variables and Constants
Swift uses var
for variables (which can change) and let
for constants (which cannot change).
var greeting = "Hello"
let name = "World"
greeting = "Hi"
print("\(greeting), \(name)!")
In this code, greeting
is a variable, so it can be changed from “Hello” to “Hi”. name
is a constant and cannot be changed.
Data Types
Swift supports various data types, including integers, floats, strings, and booleans.
let age: Int = 25
let height: Double = 5.9
let isStudent: Bool = true
let firstName: String = "John"
Functions
Functions are blocks of code that perform specific tasks. Here’s a simple function in Swift:
func greet(person: String) -> String {
return "Hello, \(person)!"
}
let message = greet(person: "Alice")
print(message)
This function greet
takes a String
parameter and returns a greeting message.
Control Flow
Swift has control flow statements like if
, for
, and while
.
let score = 85
if score >= 90 {
print("Excellent!")
} else if score >= 75 {
print("Good job!")
} else {
print("Keep trying!")
}
This code checks the value of score
and prints a corresponding message.
Arrays and Dictionaries
Arrays store ordered collections of values, while dictionaries store key-value pairs.
var fruits = ["Apple", "Banana", "Cherry"]
fruits.append("Date")
print(fruits)
var capitals = ["France": "Paris", "Japan": "Tokyo"]
capitals["Italy"] = "Rome"
print(capitals)
Classes and Structures
Classes and structures are used to create custom data types.
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func describe() -> String {
return "\(name) is \(age) years old."
}
}
let person = Person(name: "Alice", age: 30)
print(person.describe())
In this example, we define a Person
class with properties and a method to describe the person.
Swift Playgrounds
Swift Playgrounds is an iPad app that makes learning Swift fun and interactive. It’s great for beginners and provides a hands-on way to experiment with code.
It’s here PIP Python Package Manager you need to know
Conclusion
Swift is a powerful yet accessible language, perfect for developing apps in the Apple ecosystem. Its clean syntax and modern features make it an excellent choice for both beginners and experienced developers. Start with simple programs, experiment with different features, and soon you’ll be building your own apps!