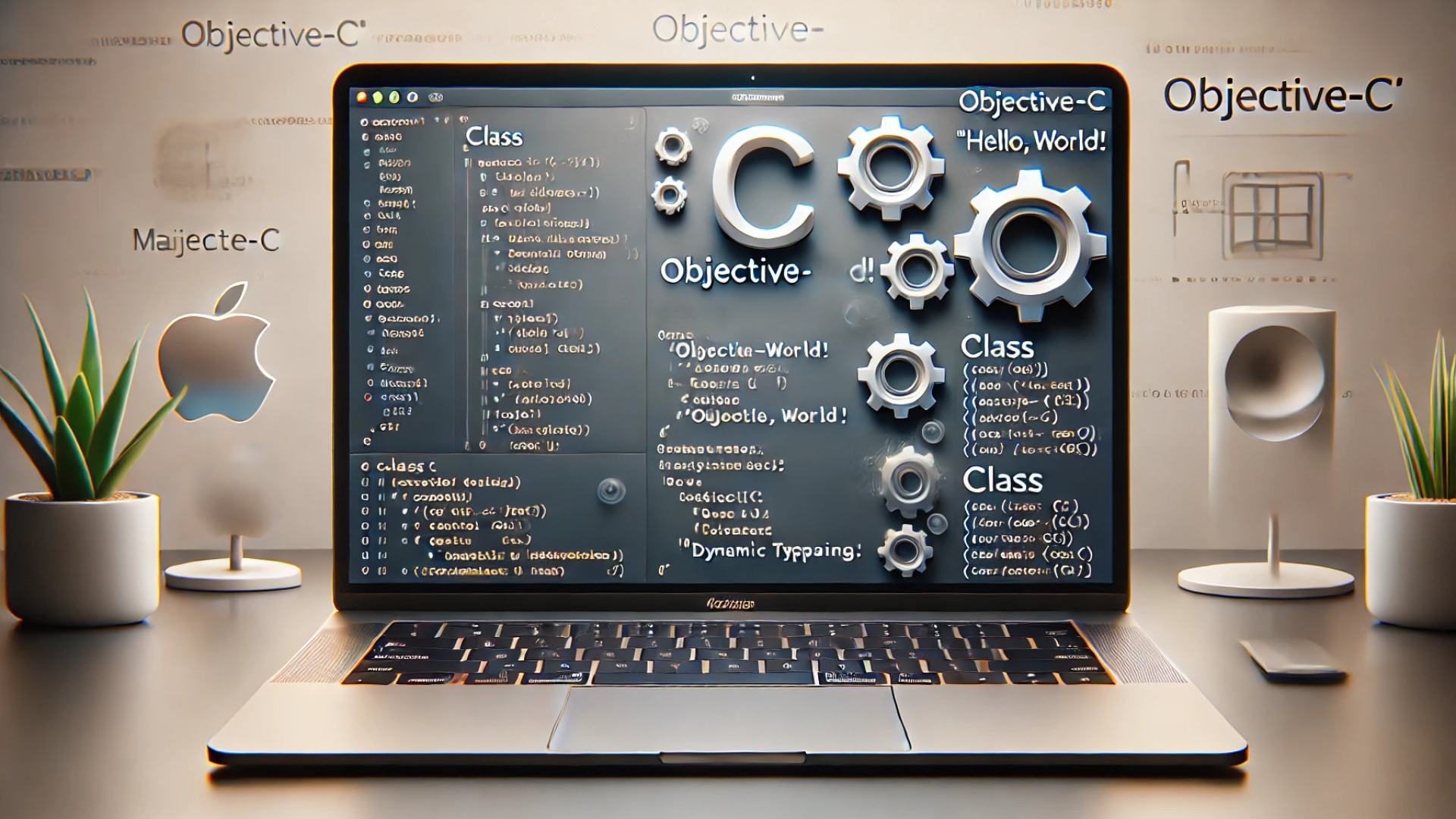
Objective-C is an object-oriented programming language that was developed in the early 1980s. It’s a superset of the C programming language, which means it can do everything C can, but with added features like object-oriented capabilities. Objective-C was the primary language used by Apple for macOS and iOS development until Swift came along in 2014.
Key Features of Objective-C
- Object-Oriented Programming (OOP): Objective-C focuses heavily on OOP, meaning it revolves around the concept of objects. These objects can hold data (in the form of properties) and behavior (in the form of methods).
- Dynamic Typing and Binding: Unlike some languages that require you to define the data type of every variable, Objective-C is dynamically typed. This flexibility allows for more dynamic and flexible code but requires careful management to avoid errors.
- Messaging: In Objective-C, instead of calling methods directly, you send messages to objects. This concept is inspired by Smalltalk, an early object-oriented language.
- Compatibility with C: Since Objective-C is a superset of C, it can use all the libraries and functions written in C. This makes it very powerful for developers who need to work with C code.
Objective-C Syntax Basics
Now, let’s dive into the basic syntax of Objective-C. If you’ve worked with C or C++, you’ll see some similarities, but Objective-C introduces its own style and structure.
1. Hello, World! in Objective-C
Let’s start with the classic “Hello, World!” example. This will give you a feel for the basic structure of an Objective-C program.
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSLog(@"Hello, World!");
}
return 0;
}
Explanation:
#import <Foundation/Foundation.h>
: This imports the Foundation framework, which provides essential classes like NSString, NSArray, and more.@autoreleasepool { ... }
: This is used to manage memory automatically. It’s important in Objective-C to prevent memory leaks.NSLog(@"Hello, World!");
: This is the Objective-C version ofprintf
in C. It logs the output to the console.
2. Classes and Objects
Objective-C is all about objects. Let’s look at how you can define a class and create an object.
#import <Foundation/Foundation.h>
@interface Person : NSObject
@property NSString *name;
@property int age;
- (void)introduceYourself;
@end
@implementation Person
- (void)introduceYourself {
NSLog(@"Hello, my name is %@ and I am %d years old.", self.name, self.age);
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
Person *person = [[Person alloc] init];
person.name = @"Alice";
person.age = 30;
[person introduceYourself];
}
return 0;
}
Explanation:
@interface
and@end
: These keywords define the structure of a class. Inside@interface
, you declare properties and methods.@property
: This automatically creates getter and setter methods for thename
andage
properties.@implementation
and@end
: This is where you implement the methods declared in the@interface
.self
: This refers to the current instance of the class.[[Person alloc] init]
: This creates a new instance of thePerson
class.
3. Memory Management
Memory management is a critical aspect of Objective-C, especially before the introduction of Automatic Reference Counting (ARC). Under ARC, most of the memory management is done automatically, but understanding the basics is still important.
- retain: Increases the reference count of an object.
- release: Decreases the reference count. When it reaches zero, the object is deallocated.
- autorelease: Marks the object for release at a later time.
Before ARC, you had to manually manage memory by retaining and releasing objects. With ARC, this is mostly handled for you, which reduces the chances of memory leaks.
Practical Examples in Objective-C
Let’s look at a few more examples to see Objective-C in action.
1. Working with Arrays
NSArray *fruits = @[@"Apple", @"Banana", @"Cherry"];
for (NSString *fruit in fruits) {
NSLog(@"%@", fruit);
}
Explanation:
NSArray
: An immutable array in Objective-C. Once created, its contents cannot be changed.@[@"Apple", @"Banana", @"Cherry"]
: This is the shorthand for creating an array.
2. Dictionary Example
NSDictionary *personInfo = @{@"name": @"John", @"age": @25};
NSString *name = [personInfo objectForKey:@"name"];
NSLog(@"Name: %@", name);
Explanation:
NSDictionary
: A collection of key-value pairs.@{@"key": @"value"}
: Shorthand for creating a dictionary.[personInfo objectForKey:@"name"]
: Retrieves the value associated with the key “name”.
Why Learn Objective-C?
You might be wondering, with Swift being so popular, why should you bother learning Objective-C? Here are a few reasons:
- Legacy Code: Many existing apps are still written in Objective-C, and understanding it is crucial if you need to maintain or update these apps.
- Interoperability: Objective-C and Swift can be used together in the same project. Knowing both languages gives you more flexibility in iOS development.
- Understanding the Foundation: Objective-C provides a deeper understanding of Apple’s frameworks and how they work under the hood.
Frequently Asked Questions (FAQs)
Q1: Is Objective-C still relevant?
Yes, especially if you’re working with legacy code or need to maintain older apps. It’s also useful to know Objective-C for a more comprehensive understanding of iOS development.
Q2: Can Objective-C and Swift be used together?
Absolutely! You can use Objective-C and Swift together in the same project, which allows you to leverage both languages’ strengths.
Q3: How hard is it to learn Objective-C compared to Swift?
Objective-C can be more challenging to learn due to its verbose syntax and concepts like manual memory management. However, once you get the hang of it, it becomes much easier.
Wrapping Up
Objective-C might not be the latest and greatest language in the Apple ecosystem, but it’s still an important one. Whether you’re diving into legacy projects or just want to broaden your programming horizons, understanding Objective-C is a valuable skill. Start with the basics, practice with simple examples, and soon enough, you’ll be writing and reading Objective-C code with confidence.