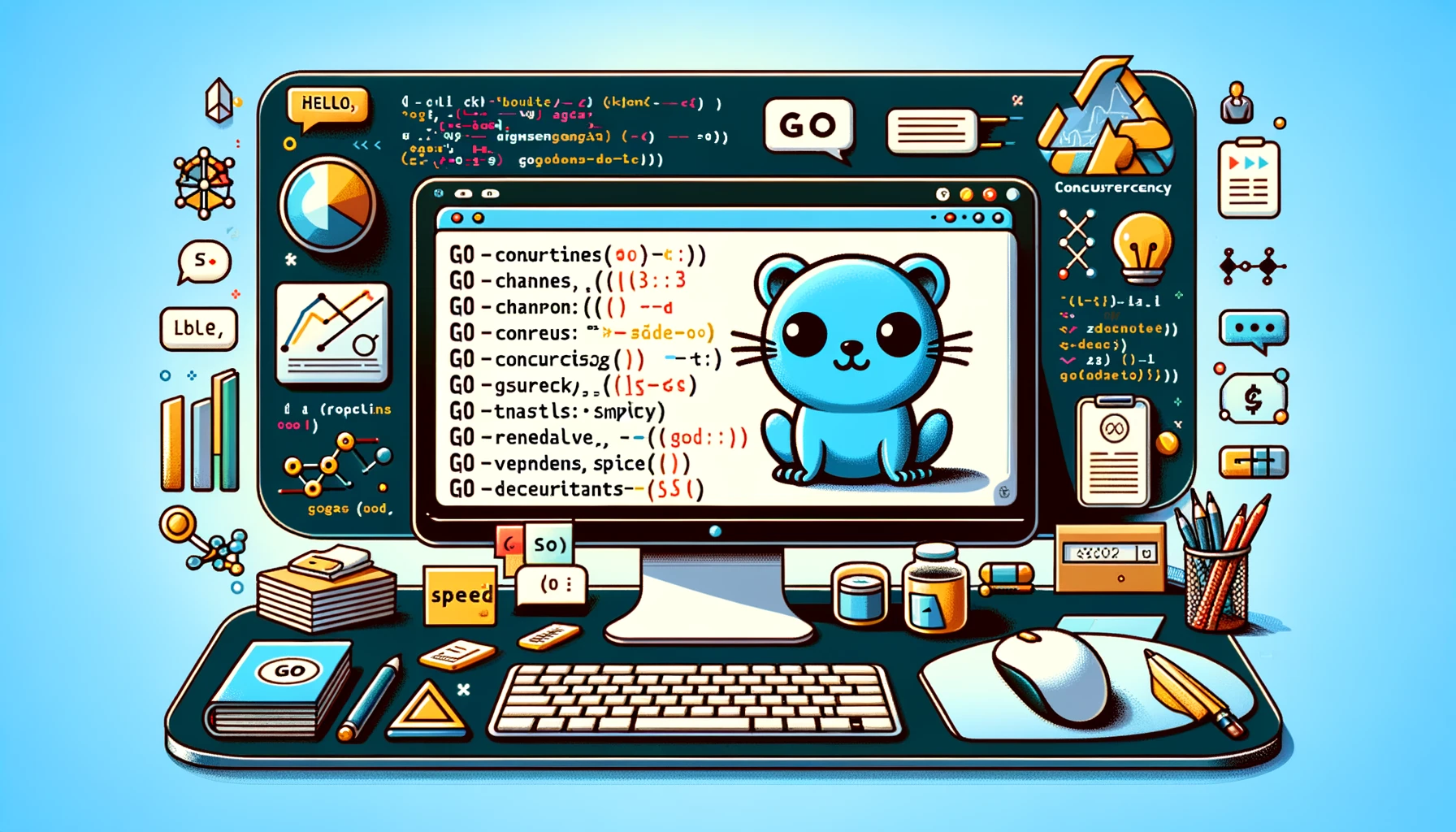
Hey there! Ready to dive into the world of Go (Golang) programming? You’re in the right place. In this guide, we’ll cover what Go is, why it’s great, and show you some neat examples to get you started. Whether you’re a newbie or looking to add another language to your toolkit, Go’s simplicity and power are sure to impress.
What is Go (Golang)?
Go, often referred to as Golang, is a programming language created by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson. It was designed to be simple, efficient, and scalable, making it perfect for modern software development.
Why Choose Go?
Go has several standout features that make it a popular choice among developers:
- Simple Syntax: Easy to learn and read, even for beginners.
- Speed: Compiled language that runs fast.
- Concurrency: Built-in support for concurrent programming.
- Scalability: Ideal for developing large, scalable systems.
- Standard Library: Rich library that covers most needs right out of the box.
Apple’s Programming Language (swift) you need to know
Getting Started with Go
First things first, you’ll need to install Go. You can download it from the official Go website. Follow the instructions for your operating system, and you’ll be ready to write your first Go program in no time.
Hello, World! in Go
Let’s start with the classic “Hello, World!” program. Open your favorite text editor and type the following:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Save the file as hello.go
. To run it, open your terminal and navigate to the directory where you saved the file. Type go run hello.go
and you should see Hello, World!
printed on the screen.
Understanding the Code
- package main: Defines the package name. A Go program starts running in the
main
package. - import “fmt”: Imports the
fmt
package, which contains functions for formatted I/O. - func main(): The main function, where the program execution begins.
- fmt.Println(“Hello, World!”): Prints the string to the console.
Variables and Types
Go is statically typed, meaning you need to declare the type of variables. Here’s an example:
package main
import "fmt"
func main() {
var name string = "Alice"
var age int = 25
var isStudent bool = true
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Is Student:", isStudent)
}
You can also use shorthand notation to declare variables:
package main
import "fmt"
func main() {
name := "Alice"
age := 25
isStudent := true
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Is Student:", isStudent)
}
Functions in Go
Functions are a key part of Go. Here’s a simple example:
package main
import "fmt"
func add(a int, b int) int {
return a + b
}
func main() {
sum := add(5, 3)
fmt.Println("Sum:", sum)
}
In this example, we define a function add
that takes two integers and returns their sum.
Control Structures
Go supports standard control structures like if-else, for loops, and switch statements. Here’s how you can use them:
package main
import "fmt"
func main() {
// If-else
age := 18
if age >= 18 {
fmt.Println("Adult")
} else {
fmt.Println("Minor")
}
// For loop
for i := 0; i < 5; i++ {
fmt.Println(i)
}
// Switch statement
day := "Monday"
switch day {
case "Monday":
fmt.Println("Start of the week")
case "Friday":
fmt.Println("End of the week")
default:
fmt.Println("Midweek day")
}
}
Concurrency in Go
One of Go’s strongest features is its support for concurrency using goroutines. Goroutines are lightweight threads managed by the Go runtime.
Here’s a simple example using goroutines and channels:
package main
import (
"fmt"
"time"
)
func say(s string) {
for i := 0; i < 5; i++ {
time.Sleep(100 * time.Millisecond)
fmt.Println(s)
}
}
func main() {
go say("Hello")
say("World")
}
In this example, say("Hello")
runs in a separate goroutine, allowing say("World")
to run concurrently.
Channels in Go
Channels are used to communicate between goroutines. Here’s a quick example:
package main
import "fmt"
func sum(a []int, c chan int) {
total := 0
for _, v := range a {
total += v
}
c <- total
}
func main() {
a := []int{1, 2, 3, 4, 5}
c := make(chan int)
go sum(a, c)
result := <-c
fmt.Println("Sum:", result)
}
In this example, a channel c
is created to send the sum of the array a
from the sum
function to the main function.
Guide to R Programming Language you need to know.
Conclusion
Go (Golang) is a powerful yet simple language that’s perfect for both beginners and seasoned developers. Its clear syntax, efficient performance, and robust standard library make it an excellent choice for a wide range of applications. Whether you’re building web servers, data pipelines, or cloud services, Go has the tools you need.
Ready to start your Go journey? Give these examples a try, and you’ll be writing your own Go programs in no time!