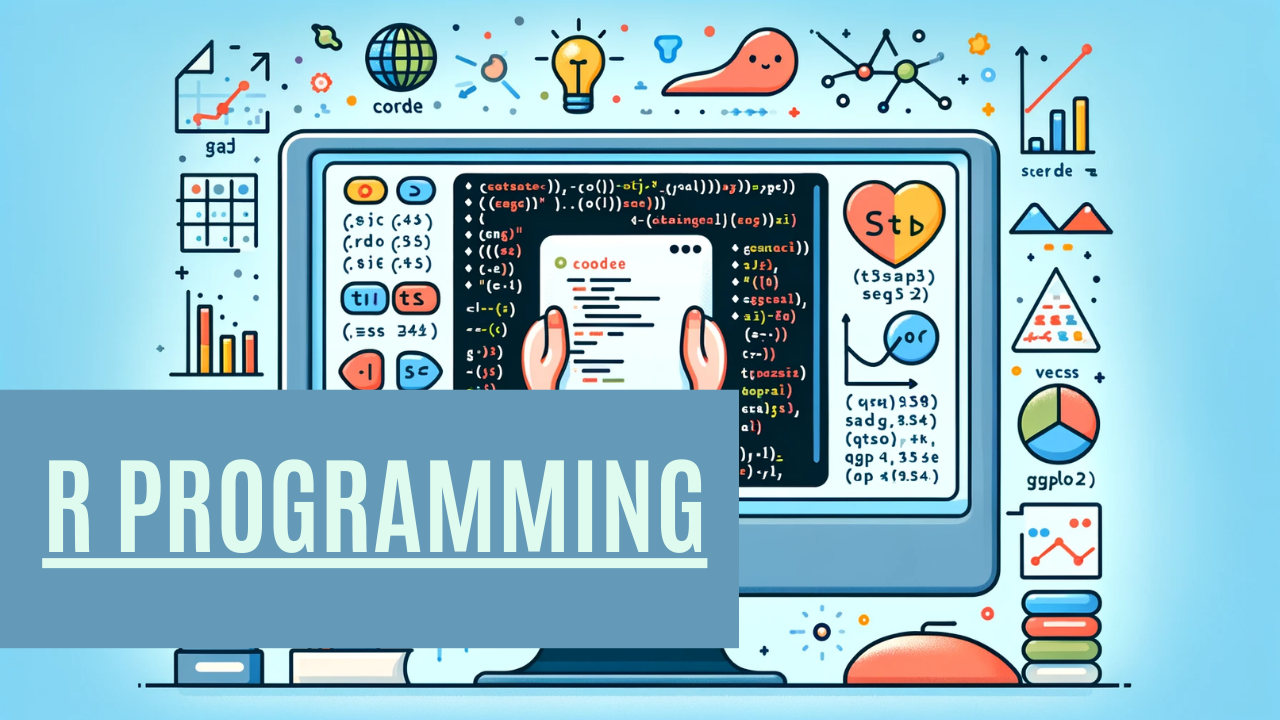
Have you ever wondered how to dive into the world of data analysis with R programming? This guide will walk you through the essentials, providing clear examples and practical tips. Whether you’re new to coding or just want to add another tool to your data analysis toolkit, R is a great place to start.
What is R Programming?
R is a programming language specifically designed for statistical computing and graphics. It’s widely used by data analysts, statisticians, and researchers to clean, analyze, and visualize data. One of the coolest things about R is its powerful and extensive collection of packages, which are like add-ons that extend the language’s capabilities.
Getting Started with R
First things first, you’ll need to install R and RStudio. RStudio is an integrated development environment (IDE) for R, making it easier to write and manage your R scripts.
- Download R: Visit the CRAN website and choose the version that matches your operating system.
- Install RStudio: Go to the RStudio website and download the free version.
Once you have both installed, open RStudio, and you’ll see a user-friendly interface where you can start coding.
Basic Syntax and Commands
Let’s dive into some basic R commands to get you started.
Creating Variables
In R, you can create variables to store data. Here’s how:
# Assigning a number to a variable
x <- 5
# Assigning a string to a variable
name <- "R Programming"
# Assigning a list to a variable
numbers <- c(1, 2, 3, 4, 5)
Basic Arithmetic Operations
You can perform basic arithmetic operations like addition, subtraction, multiplication, and division.
# Addition
sum <- 2 + 3
# Subtraction
difference <- 5 - 2
# Multiplication
product <- 3 * 4
# Division
quotient <- 10 / 2
Functions in R
Functions are used to perform specific tasks. R has many built-in functions, and you can also create your own.
# Built-in function: sqrt() to calculate the square root
sqrt_16 <- sqrt(16)
# Creating a custom function
add_numbers <- function(a, b) {
return(a + b)
}
# Using the custom function
result <- add_numbers(3, 7)
Data Structures in R
R has several data structures to store and manipulate data. The most common ones are vectors, matrices, data frames, and lists.
Vectors
Vectors are one-dimensional arrays that can hold numeric, character, or logical data.
# Numeric vector
num_vector <- c(1, 2, 3, 4, 5)
# Character vector
char_vector <- c("apple", "banana", "cherry")
# Logical vector
log_vector <- c(TRUE, FALSE, TRUE)
Matrices
Matrices are two-dimensional, homogeneous data structures.
# Creating a matrix
matrix_data <- matrix(1:9, nrow = 3, ncol = 3)
Data Frames
Data frames are like tables or spreadsheets, where each column can contain different types of data.
# Creating a data frame
data_frame <- data.frame(
name = c("John", "Doe", "Anna"),
age = c(28, 34, 22),
score = c(85, 90, 88)
)
Lists
Lists can hold different types of elements, including vectors, matrices, data frames, and even other lists.
# Creating a list
list_data <- list(
name = "R Language",
numbers = num_vector,
matrix = matrix_data
)
Data Analysis and Visualization
R is incredibly powerful for data analysis and visualization. Let’s explore some basic data manipulation and plotting.
Loading Data
You can load data from various sources, including CSV files.
# Reading a CSV file
data <- read.csv("path/to/your/data.csv")
Basic Data Manipulation
You can filter, sort, and summarize data easily in R.
# Filtering data
filtered_data <- subset(data, age > 25)
# Sorting data
sorted_data <- data[order(data$score), ]
# Summarizing data
summary(data)
Data Visualization with ggplot2
One of the most popular packages for data visualization in R is ggplot2
. Here’s a simple example.
# Installing ggplot2 package
install.packages("ggplot2")
# Loading ggplot2 library
library(ggplot2)
# Creating a basic scatter plot
ggplot(data, aes(x = age, y = score)) +
geom_point()
RealWorld Use of Java Programming you need to know
Conclusion
R programming is a fantastic tool for anyone looking to dive into data analysis and visualization. With its vast array of packages and capabilities, it offers endless possibilities for working with data. By starting with the basics and gradually exploring more advanced topics, you can harness the full power of R in your projects.