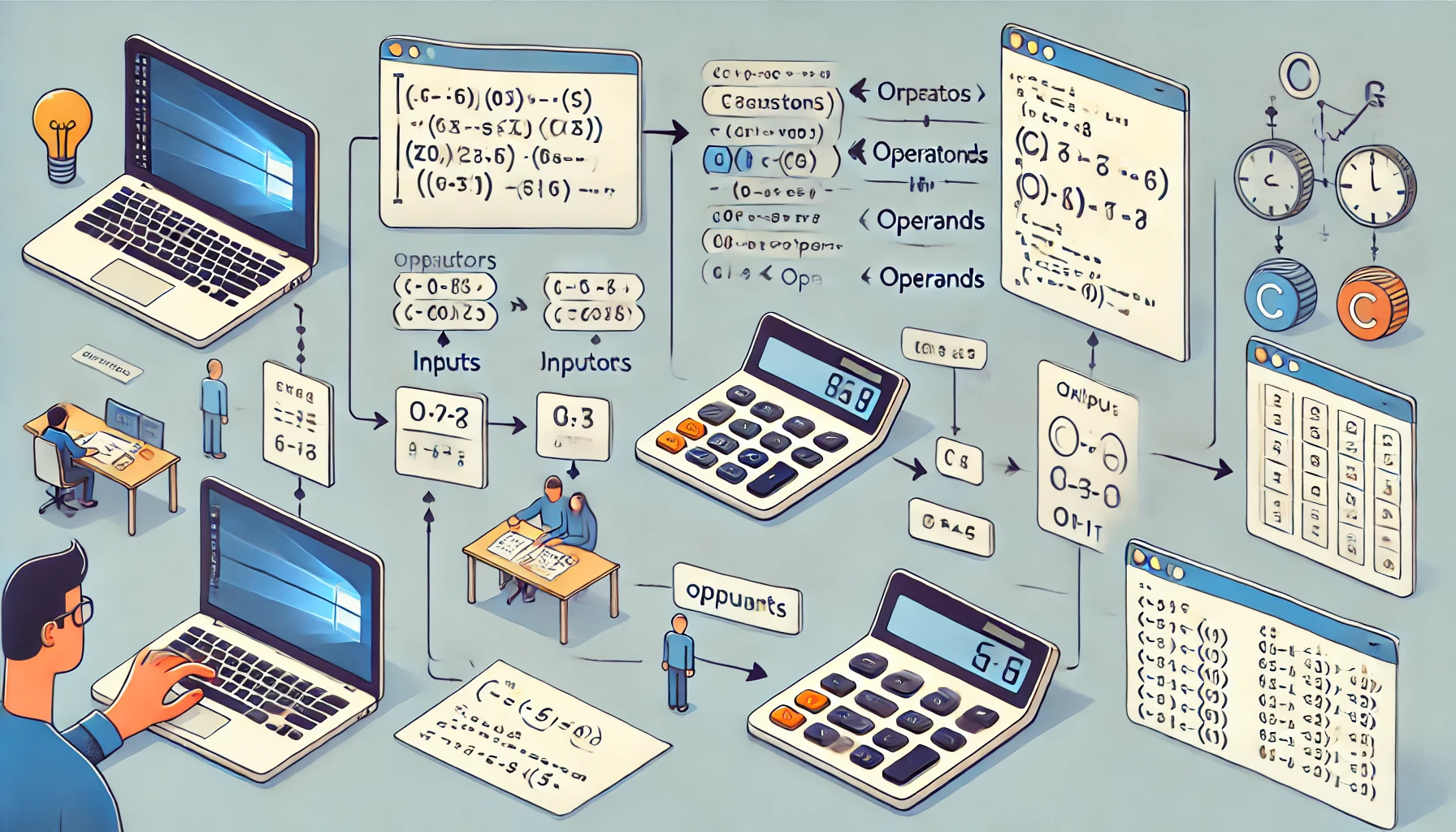
Creating an arithmetic calculator in C is a great way to sharpen your programming skills. This guide will walk you through the process step-by-step, providing example code and output to help you understand the concepts clearly. Whether you’re a beginner or looking to refresh your knowledge, this tutorial is for you.
In this tutorial, you’ll learn how to create a simple arithmetic calculator using the C programming language. We will cover the basic operations of addition, subtraction, multiplication, and division. By the end, you’ll have a fully functional calculator that takes user input and performs these operations.
What We’ll Cover
- Setting up the development environment
- Writing the C code for the calculator
- Understanding the code step-by-step
- Example code and expected output
Setting Up Your Development Environment
Before diving into the code, make sure you have a C compiler installed. If you don’t have one, you can download GCC (GNU Compiler Collection) which is widely used. For Windows users, installing MinGW is a good option, and macOS/Linux users can use their respective package managers.
Writing the C Code for the Calculator
Let’s start by writing the code for our arithmetic calculator. Open your favorite text editor or IDE and type the following code:
#include <stdio.h>
int main() {
char operator;
double firstNumber, secondNumber;
printf("Enter an operator (+, -, *, /): ");
scanf("%c", &operator);
printf("Enter two operands: ");
scanf("%lf %lf", &firstNumber, &secondNumber);
switch (operator) {
case '+':
printf("%.2lf + %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber + secondNumber);
break;
case '-':
printf("%.2lf - %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber - secondNumber);
break;
case '*':
printf("%.2lf * %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber * secondNumber);
break;
case '/':
if (secondNumber != 0.0)
printf("%.2lf / %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber / secondNumber);
else
printf("Error! Division by zero.\n");
break;
default:
printf("Error! Operator is not correct\n");
break;
}
return 0;
}
it’s here C++ vs java has to know.
Understanding the Code Step-by-Step
- Including the Standard Input Output Library:
#include <stdio.h>
This line includes the standard input-output library which allows us to use functions like printf
and scanf
.
- Defining the
main
Function:
int main() {
The main
function is the entry point of the program.
- Declaring Variables:
char operator;
double firstNumber, secondNumber;
We declare a character variable operator
to store the operator and two double variables to store the operands.
- Taking User Input for Operator:
printf("Enter an operator (+, -, *, /): ");
scanf("%c", &operator);
We prompt the user to enter an operator and store it in the operator
variable.
- Taking User Input for Operands:
printf("Enter two operands: ");
scanf("%lf %lf", &firstNumber, &secondNumber);
We prompt the user to enter two operands and store them in firstNumber
and secondNumber
.
- Using Switch Case to Perform Operations:
switch (operator) {
case '+':
printf("%.2lf + %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber + secondNumber);
break;
case '-':
printf("%.2lf - %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber - secondNumber);
break;
case '*':
printf("%.2lf * %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber * secondNumber);
break;
case '/':
if (secondNumber != 0.0)
printf("%.2lf / %.2lf = %.2lf\n", firstNumber, secondNumber, firstNumber / secondNumber);
else
printf("Error! Division by zero.\n");
break;
default:
printf("Error! Operator is not correct\n");
break;
}
We use a switch case statement to perform the operation based on the operator entered by the user. If the user enters an invalid operator or tries to divide by zero, we print an error message.
- Returning 0 to Indicate Successful Execution:
return 0;
This line indicates that the program has executed successfully.
It’s here Data Structures in C++ need to know.
Example Output
Let’s see how the program works with some example inputs:
Example 1
Enter an operator (+, -, *, /): +
Enter two operands: 5 3
5.00 + 3.00 = 8.00
Example 2
Enter an operator (+, -, *, /): /
Enter two operands: 10 2
10.00 / 2.00 = 5.00
Example 3
Enter an operator (+, -, *, /): /
Enter two operands: 10 0
Error! Division by zero.
Conclusion
Congratulations! You’ve successfully created a simple arithmetic calculator in C. This basic project helps you understand user input, conditional statements, and basic arithmetic operations in C. Keep experimenting with the code and try adding more features like handling more operators or performing multiple operations in a single run.
Feel free to share your thoughts or ask questions in the comments below. Happy coding!