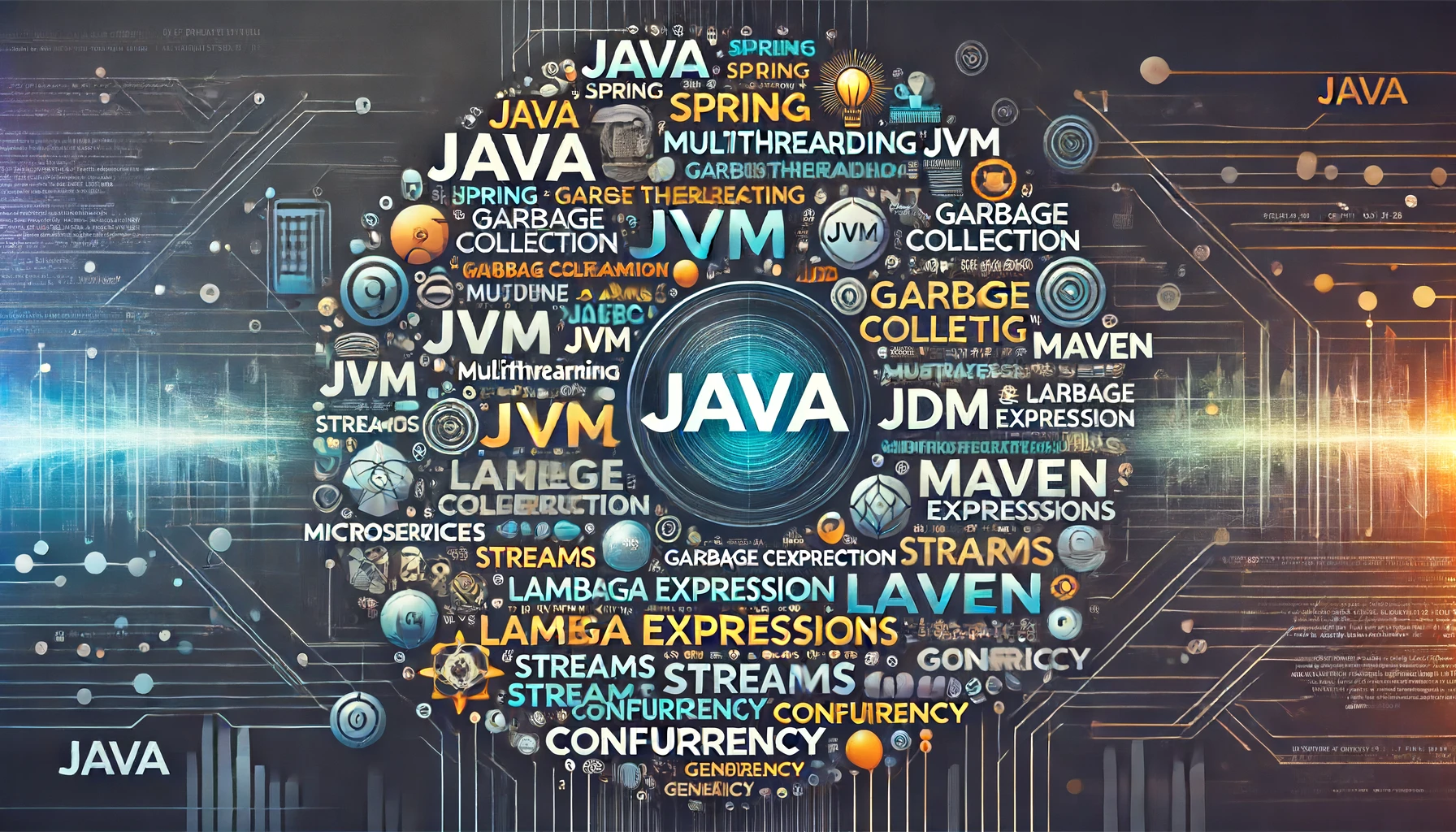
Java is a powerhouse in the programming world, known for its robustness, security, and portability. However, diving into Java can be overwhelming due to the numerous terms and buzzwords thrown around. Understanding these buzzwords isn’t just about speaking the lingo; it’s about grasping the core concepts that make Java so effective. In this blog, we’ll explore the importance of key Java buzzwords and provide examples to illustrate their practical applications.
Why Are Java Buzzwords Important?
Java buzzwords are more than just jargon. They encapsulate essential features and principles of the language that every programmer should understand. Knowing these buzzwords helps you write better code, debug more efficiently, and communicate more effectively with other developers.
Key Java Buzzwords and Their Significance
1. Platform Independence
Definition: Java’s ability to run the same bytecode on any device that has a Java Virtual Machine (JVM) installed.
Significance: This is a core feature that makes Java a “write once, run anywhere” language. It ensures that Java applications are portable and can operate across various platforms without modification.
Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This simple program can run on any operating system with a JVM, demonstrating Java’s platform independence.
2. Object-Oriented
Definition: Java is an object-oriented programming (OOP) language, which means it is based on objects and classes.
Significance: OOP principles help in organizing complex programs, making them more modular, flexible, and easy to maintain. Key OOP concepts include inheritance, encapsulation, polymorphism, and abstraction.
Example:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Dog barks");
}
}
public class Test {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound(); // Outputs: Dog barks
}
}
Here, Dog
inherits from Animal
, demonstrating inheritance and polymorphism.
3. Robust
Definition: Java is designed to be robust, with strong memory management, exception handling, and garbage collection.
Significance: Robustness ensures that Java programs are less prone to crashes and errors. The language’s built-in mechanisms handle runtime errors efficiently, enhancing reliability.
Example:
public class RobustExample {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[10]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array index is out of bounds!");
}
}
}
This example shows exception handling, a key aspect of Java’s robustness.
4. Multithreading
Definition: Java supports multithreading, allowing concurrent execution of two or more threads.
Significance: Multithreading improves the performance of applications, especially those that require significant computational resources, by executing tasks simultaneously.
Example:
class MyThread extends Thread {
public void run() {
for (int i = 1; i <= 5; i++) {
System.out.println(i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println(e);
}
}
}
public static void main(String[] args) {
MyThread t1 = new MyThread();
MyThread t2 = new MyThread();
t1.start();
t2.start();
}
}
This program creates two threads that run concurrently, demonstrating Java’s multithreading capabilities.
5. Secure
Definition: Java has a comprehensive security framework that ensures a secure execution environment.
Significance: Security features in Java, such as bytecode verification and the Security Manager, protect against malicious code and unauthorized access, making Java a preferred choice for internet-based applications.
Example:
import java.security.*;
public class SecurityExample {
public static void main(String[] args) {
SecurityManager sm = new SecurityManager();
System.setSecurityManager(sm);
try {
System.getProperty("user.home");
} catch (SecurityException se) {
System.out.println("Access denied!");
}
}
}
This example demonstrates the use of a Security Manager to enforce a security policy.
useful datatypes in java that you don’t know.
Conclusion: Embrace Java Buzzwords
Understanding and applying Java buzzwords is crucial for any developer aiming to master the language. These concepts not only enhance your coding skills but also enable you to create efficient, secure, and scalable applications. So, the next time you come across a Java buzzword, embrace it, and consider how it can improve your programming practices.