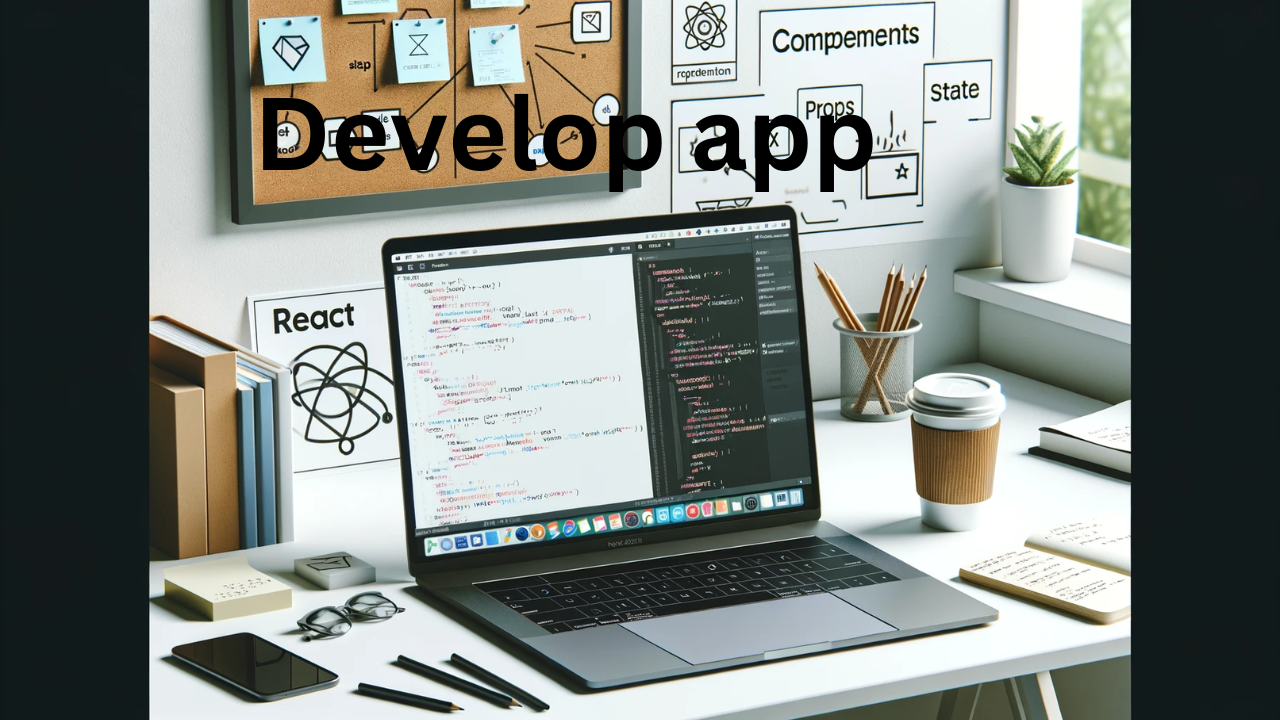
React is a popular JavaScript library for building user interfaces, especially for single-page applications. It allows developers to create large web applications that can update and render efficiently in response to data changes. Whether you’re a seasoned developer or just starting, this guide will help you understand React’s core concepts with simple words and practical examples.
What is React?
React, developed by Facebook, is an open-source JavaScript library. It focuses on building user interfaces (UIs) and is particularly useful for creating single-page applications where you need a dynamic, fast, and interactive user experience.
Why Use React?
React is popular for several reasons:
- Component-Based Architecture: Breaks the UI into reusable components.
- Virtual DOM: Efficient updates and rendering.
- One-Way Data Binding: Makes code more predictable and easier to debug.
- Strong Community and Ecosystem: Extensive libraries, tools, and community support.
Guide to R Programming Language you need to know.
Getting Started with React
Before diving in, ensure you have Node.js and npm (Node Package Manager) installed on your computer. You can download them from nodejs.org.
Step 1: Setting Up Your Environment
- Open your terminal or command prompt.
- Use the following command to install
create-react-app
, a tool to set up a new React project:
npx create-react-app my-first-react-app
- Navigate into your project directory:
cd my-first-react-app
- Start the development server:
npm start
Your default browser should open, displaying your new React app at http://localhost:3000/
.
Understanding React Components
Components are the building blocks of a React application. There are two types of components: functional and class components.
Functional Components
Functional components are simple JavaScript functions that return JSX (JavaScript XML). They are easy to read and write.
Example:
import React from 'react';
function Greeting() {
return <h1>Hello, world!</h1>;
}
export default Greeting;
Class Components
Class components are ES6 classes that extend from React.Component
. They have more features, such as state and lifecycle methods.
Example:
import React, { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, world!</h1>;
}
}
export default Greeting;
JSX: JavaScript XML
JSX is a syntax extension for JavaScript. It allows you to write HTML directly within React. Browsers don’t understand JSX out of the box, so it gets transformed into regular JavaScript by tools like Babel.
Example:
const element = <h1>Hello, world!</h1>;
This gets converted to:
const element = React.createElement('h1', null, 'Hello, world!');
Props and State
Props
Props (short for properties) are read-only inputs passed to components. They help make components dynamic and reusable.
Example:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name="Sara" />;
State
State is a built-in object that holds data or information about the component. Unlike props, state is managed within the component.
Example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
Handling Events
React handles events in a way similar to handling events in HTML, but with some syntax differences.
Example:
function ActionLink() {
function handleClick(e) {
e.preventDefault();
console.log('The link was clicked.');
}
return (
<a href="#" onClick={handleClick}>
Click me
</a>
);
}
It’s here PIP Python Package Manager you need to know
Conclusion
React simplifies the process of building dynamic and interactive UIs with its component-based architecture and efficient rendering capabilities. By understanding the basics of components, JSX, props, state, and event handling, you’re well on your way to mastering React programming.
Remember, the key to becoming proficient in React is practice. Start building small projects, explore the rich ecosystem of libraries and tools, and soon enough, you’ll be creating complex applications with ease.