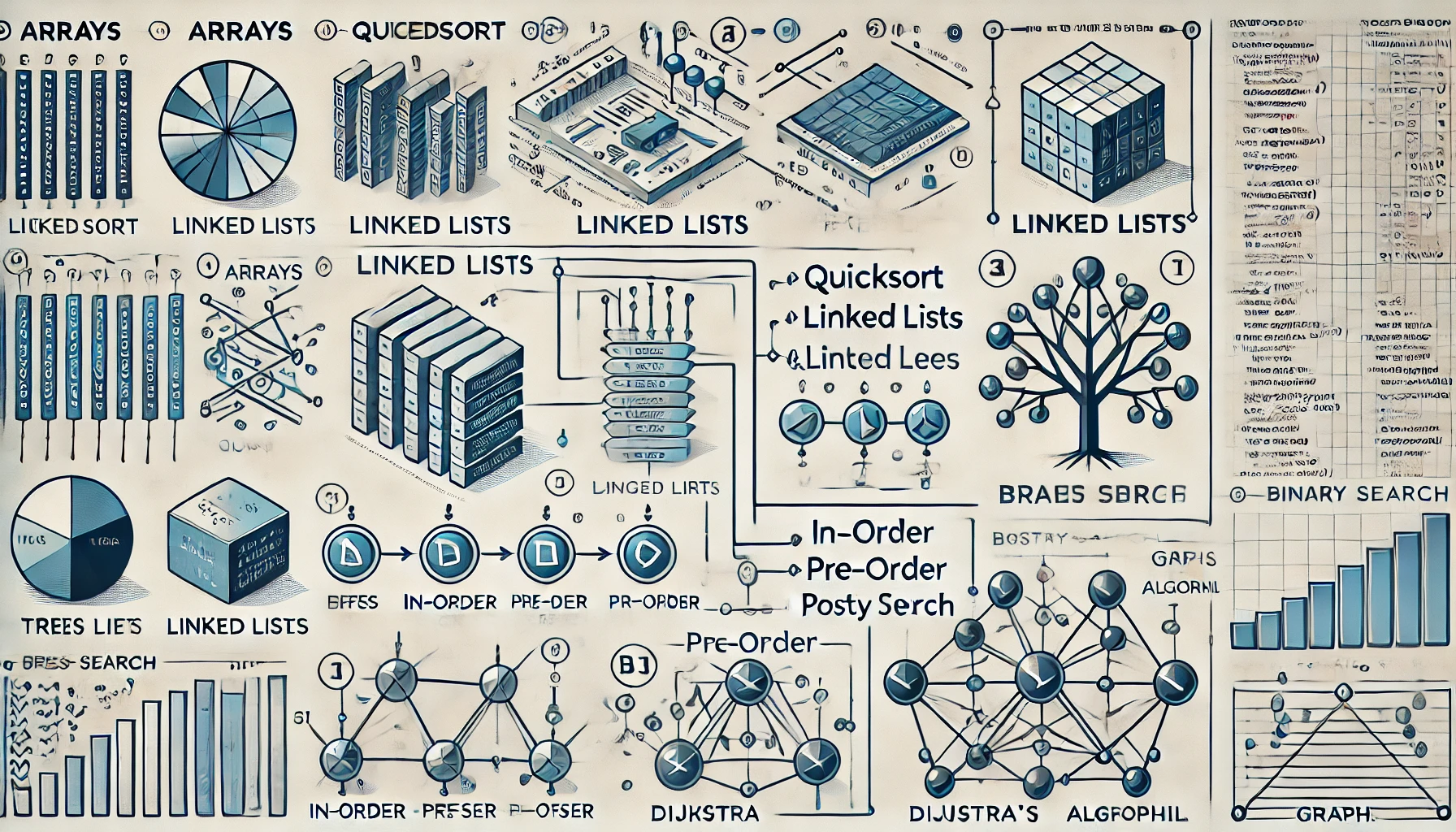
In today’s data-driven world, algorithms are the backbone of efficient data processing and storage. Whether you’re sorting an array or traversing a graph, understanding the right algorithms for each data type is crucial. This guide will walk you through essential algorithms for some of the most important data types: arrays, linked lists, trees, and graphs. Let’s get started!
Arrays: The Building Blocks
Arrays are the simplest and most commonly used data structures. They store elements in contiguous memory locations, which makes accessing elements by index extremely fast. However, certain operations can be slow if not optimized with the right algorithms.
Sorting Algorithms
- QuickSort: This is a highly efficient sorting algorithm with an average-case time complexity of O(n log n). It works by selecting a ‘pivot’ element and partitioning the array into two halves.
- MergeSort: Another efficient algorithm with a guaranteed time complexity of O(n log n). It divides the array into halves, recursively sorts them, and then merges them back together.
- BubbleSort: Simple but less efficient, with a time complexity of O(n^2). It repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order.
Search Algorithms
- Binary Search: This algorithm is efficient for searching sorted arrays, with a time complexity of O(log n). It repeatedly divides the search interval in half.
- Linear Search: Although not as efficient, with a time complexity of O(n), it is straightforward and works on both sorted and unsorted arrays.
Linked Lists: Flexibility in Structure
Linked lists consist of nodes where each node contains data and a reference to the next node. They provide flexibility over arrays but come with different algorithmic needs.
Traversal Algorithms
- Iterative Traversal: Simply iterates through the list node by node until the end is reached.
- Recursive Traversal: Uses the call stack to traverse the list, which can be more elegant but may risk stack overflow for very long lists.
Insertion and Deletion
- Insertion at Beginning: Inserting a node at the beginning of the list is an O(1) operation.
- Insertion at End: Requires traversing the list to the end, making it an O(n) operation.
- Deletion: Deleting a node can also vary in complexity. Deleting the first node is O(1), but deleting a node at a specific position is O(n) because it requires traversal.
Trees: Hierarchical Data Handling
Trees are hierarchical structures with nodes that have children. The most common type is the binary tree, where each node has at most two children.
Traversal Algorithms
- In-Order Traversal: Visits nodes in a left-root-right order. It’s used for binary search trees to retrieve data in sorted order.
- Pre-Order Traversal: Visits nodes in a root-left-right order. Useful for copying trees.
- Post-Order Traversal: Visits nodes in a left-right-root order. It’s used to delete trees.
Search Algorithms
- Binary Search Tree (BST): A tree where each node has at most two children, and the left child is less than the parent node, while the right child is greater. Searching in a BST is O(h), where h is the height of the tree.
- AVL Tree: A self-balancing binary search tree, which maintains an O(log n) search time by ensuring the tree remains balanced.
Graphs: Complex Relationships and Pathfinding
Graphs consist of vertices (nodes) and edges (connections between nodes). They model complex relationships and require sophisticated algorithms.
Traversal Algorithms
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking. Uses a stack data structure.
- Breadth-First Search (BFS): Explores all neighbors at the present depth before moving on to nodes at the next depth level. Uses a queue data structure.
Pathfinding Algorithms
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a weighted graph. It uses a priority queue and has a time complexity of O(V^2) or O(E + V log V) with a binary heap.
- A Algorithm:* An extension of Dijkstra’s that uses heuristics to improve performance. It’s commonly used in AI and game development.
Minimum Spanning Tree Algorithms
- Kruskal’s Algorithm: Finds the minimum spanning tree by sorting edges and adding them one by one, ensuring no cycles form. It uses a union-find data structure.
- Prim’s Algorithm: Builds the minimum spanning tree by starting with a single vertex and adding the cheapest edge from the tree to a vertex outside the tree.
How Coding Algorithms works you need to know
Wrapping It Up
Understanding these essential algorithms for different data types is vital for optimizing data processing and storage. Whether you’re dealing with arrays, linked lists, trees, or graphs, the right algorithm can make a world of difference in performance and efficiency.
Got questions about algorithms or need further clarification on any of the points mentioned? Feel free to ask!