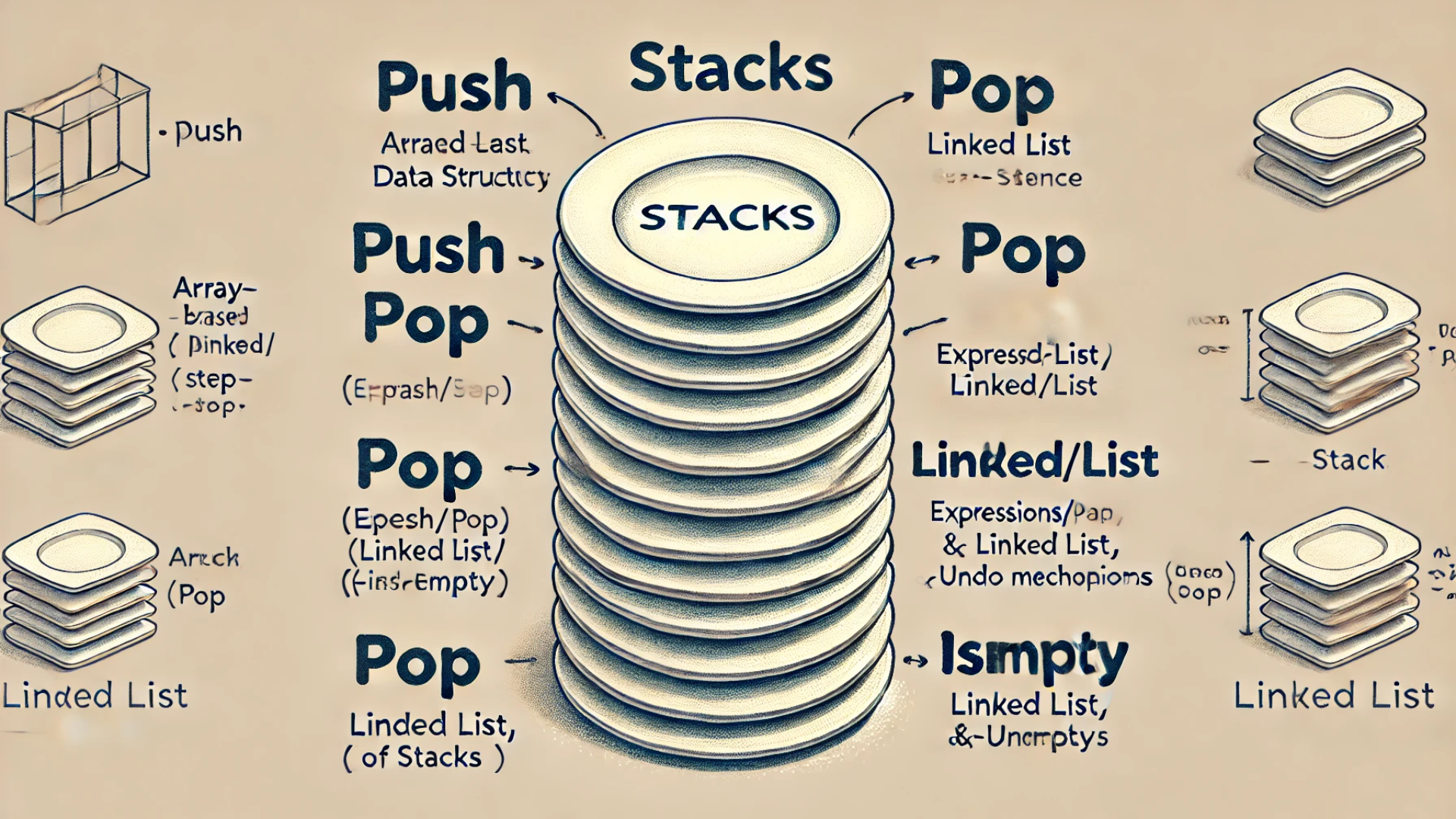
Stacks are a fundamental concept in computer science, often compared to a stack of plates. You add and remove plates from the top, right? Stacks work the same way. Let’s explore what stacks are, how they function, and why they’re important.
What is a Stack?
A stack is a linear data structure that follows a particular order in which operations are performed. The order may be LIFO (Last In, First Out) or FILO (First In, Last Out). Here’s a quick rundown:
- LIFO (Last In, First Out): The last element added to the stack will be the first one to be removed.
- FILO (First In, Last Out): The first element added will be the last one removed. Essentially, it’s the same as LIFO from a conceptual standpoint.
Basic Operations of a Stack
Stacks have four primary operations:
- Push: Adds an item to the stack. If the stack is full, it’s called an overflow condition.
- Pop: Removes the most recently added item. If the stack is empty, it’s called an underflow condition.
- Peek/Top: Returns the top item without removing it.
- IsEmpty: Checks if the stack is empty.
Stack Representation
Stacks can be implemented using arrays or linked lists. Here’s a basic idea:
Array Representation
In an array-based stack, a single-dimensional array is used. A variable called top
is used to track the top element of the stack.
class Stack:
def __init__(self):
self.stack = []
self.top = -1
def push(self, item):
self.stack.append(item)
self.top += 1
def pop(self):
if self.is_empty():
return "Stack Underflow"
item = self.stack.pop()
self.top -= 1
return item
def peek(self):
if self.is_empty():
return "Stack is Empty"
return self.stack[self.top]
def is_empty(self):
return self.top == -1
Linked List Representation
In a linked list-based stack, each node contains data and a reference to the next node. The top of the stack is the head of the linked list.
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Stack:
def __init__(self):
self.top = None
def push(self, item):
new_node = Node(item)
new_node.next = self.top
self.top = new_node
def pop(self):
if self.is_empty():
return "Stack Underflow"
item = self.top.data
self.top = self.top.next
return item
def peek(self):
if self.is_empty():
return "Stack is Empty"
return self.top.data
def is_empty(self):
return self.top is None
Real-World Applications of Stacks
Stacks are used in various applications due to their LIFO nature. Some common uses include:
- Expression Evaluation: Stacks are used to evaluate expressions, especially postfix and prefix expressions.
- Function Calls: In many programming languages, the function calls are managed using stacks. Each time a function is called, its data is pushed onto the stack, and when it returns, the data is popped off.
- Undo Mechanism: Applications like text editors use stacks to keep track of the user’s actions, allowing undo and redo operations.
Example: Evaluating Postfix Expressions
Postfix notation (also known as Reverse Polish Notation) is where the operator follows the operands. For example, the expression “3 4 + 2 *” is equivalent to (3 + 4) * 2.
Here’s how a stack can evaluate a postfix expression:
def evaluate_postfix(expression):
stack = Stack()
for char in expression.split():
if char.isdigit():
stack.push(int(char))
else:
val1 = stack.pop()
val2 = stack.pop()
if char == '+':
stack.push(val2 + val1)
elif char == '-':
stack.push(val2 - val1)
elif char == '*':
stack.push(val2 * val1)
elif char == '/':
stack.push(val2 / val1)
return stack.pop()
expression = "3 4 + 2 *"
print("Postfix Evaluation:", evaluate_postfix(expression))
It’s here Power of Algorithms for Key Data Types
Advantages and Disadvantages of Using Stacks
Advantages
- Simplicity: Stacks are straightforward to implement and use.
- Memory Management: Automatic management of memory through stack allocation.
Disadvantages
- Limited Access: Elements can only be accessed in a specific order.
- Size Constraints: In array-based stacks, a predefined size can lead to overflow.
Conclusion
Stacks are a vital part of computer science and programming, offering a simple yet powerful way to manage data. Whether you’re evaluating expressions, managing function calls, or implementing undo features, understanding how stacks work is crucial. By mastering stack operations and their implementations, you’ll gain a valuable tool for solving various computational problems.