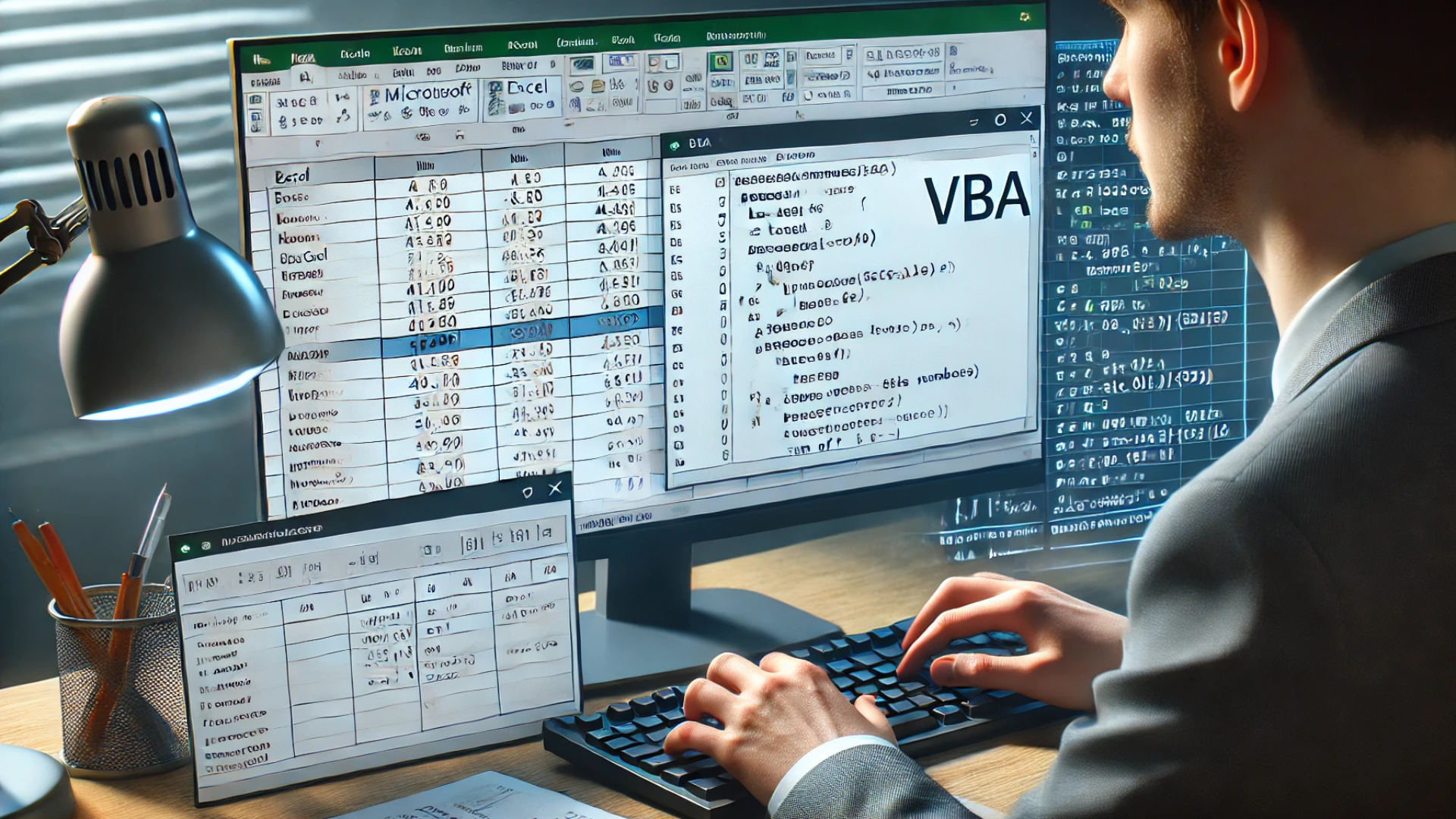
VBA (Visual Basic for Applications) is a powerful tool within Microsoft Office that can save you tons of time by automating repetitive tasks. If you’ve ever felt bogged down by doing the same thing over and over in Excel, Word, or Access, VBA is the solution you need. In this guide, we’ll break down the basics of VBA in simple terms, so you can start using it confidently.
What is VBA?
VBA, or Visual Basic for Applications, is a programming language developed by Microsoft. It’s built into Office programs like Excel, Word, and Access, allowing users to automate tasks, create custom functions, and even develop their own tools or add-ins. Think of it as a “behind-the-scenes” worker that can handle repetitive actions or complex calculations with just a few lines of code.
For instance, instead of manually copying and pasting data from one Excel sheet to another every day, you could write a small VBA script that does it for you in seconds!
Why Learn VBA?
Before we dive into the code, let’s take a quick look at the benefits of learning VBA:
- Save Time: Automate repetitive tasks to free up your time for more important work.
- Improve Accuracy: Reduce human error by letting VBA handle data processing.
- Boost Efficiency: Speed up workflows by creating your own shortcuts and tools.
- Customization: Tailor Office applications like Excel to your specific needs with custom macros and functions.
Key Components of VBA
Now that you know why VBA is so useful, let’s get familiar with some key terms and components. If you’ve never coded before, don’t worry! We’ll keep it simple.
1. Macro
A macro is a sequence of instructions that can be executed automatically. In VBA, macros are essentially small programs you write to perform tasks in Office applications. You can record a macro by performing the task manually, and VBA will automatically generate the code for you.
2. Subroutine (Sub)
A subroutine is a chunk of VBA code that performs a specific task. You can think of it as a set of instructions you give VBA. Every macro is a subroutine. Here’s a basic example of a subroutine in VBA:
Sub SayHello()
MsgBox "Hello, World!"
End Sub
When you run this subroutine, a pop-up message box will display “Hello, World!” Simple, right?
3. Variables
Just like in math, variables in VBA store data. You can store numbers, text, or even the results of calculations in variables. Here’s how you can declare a variable and use it in VBA:
Sub ShowVariable()
Dim myMessage As String
myMessage = "VBA is cool!"
MsgBox myMessage
End Sub
In this example, myMessage
is a variable that holds the text “VBA is cool!”, and the MsgBox
displays that message to the user.
4. Functions
A function in VBA is like a mini-program that returns a result. You can either use built-in functions (like SUM, AVERAGE, etc.) or create your own custom functions. Here’s an example of a simple custom function that adds two numbers:
Function AddNumbers(num1 As Integer, num2 As Integer) As Integer
AddNumbers = num1 + num2
End Function
You can call this function anywhere in your VBA code to add two numbers.
How to Write Your First VBA Code in Excel
Enough theory! Let’s jump into an example so you can see how it all works. We’ll create a simple macro that adds values from two cells in Excel and displays the result in a message box.
Step 1: Open the VBA Editor
- Open Excel.
- Press
Alt + F11
to open the VBA Editor (also called the Visual Basic Editor or VBE). - In the editor, click on Insert in the top menu and select Module. This will create a new code module where you can write your VBA code.
Step 2: Write Your First VBA Code
Now, paste the following code into the module:
Sub AddCells()
Dim cell1 As Integer
Dim cell2 As Integer
Dim result As Integer
' Get values from cells A1 and A2
cell1 = Range("A1").Value
cell2 = Range("A2").Value
' Add the values together
result = cell1 + cell2
' Show the result in a message box
MsgBox "The sum of the two cells is " & result
End Sub
This script does the following:
- Declares three variables (
cell1
,cell2
, andresult
). - Retrieves the values from cells A1 and A2.
- Adds those values together and stores the sum in the
result
variable. - Displays the result in a pop-up message box.
Step 3: Run Your Macro
- Close the VBA editor.
- In Excel, press
Alt + F8
to open the Macro dialog box. - Select the
AddCells
macro from the list and click Run.
Make sure you have numbers in cells A1 and A2, and when you run the macro, it will pop up a message showing their sum!
Common VBA Functions and Techniques
Once you’ve got the hang of writing basic macros, you can start exploring more advanced VBA techniques. Here are a few handy tips:
- Loops: VBA allows you to repeat tasks with loops. For example, if you need to apply the same action to every cell in a column, you can use a loop:
Sub LoopThroughCells()
Dim i As Integer
For i = 1 To 10
Cells(i, 1).Value = i
Next i
End Sub
This loop will fill the first column with numbers 1 through 10.
- Conditional Statements: Use
If-Else
statements to perform actions based on conditions:
Sub CheckValue()
Dim num As Integer
num = Range("A1").Value
If num > 10 Then
MsgBox "The number is greater than 10"
Else
MsgBox "The number is 10 or less"
End If
End Sub
FAQs
Q1: Is VBA difficult to learn?
Not at all! VBA is quite beginner-friendly, especially if you’re familiar with Excel. With some practice, you can automate many tasks in no time.
Q2: Can I use VBA in programs other than Excel?
Yes! VBA is also available in Word, Access, PowerPoint, and other Microsoft Office applications.
Q3: Do I need to be a programmer to use VBA?
Nope. While VBA is a programming language, you don’t need to be a full-fledged programmer to start using it. With some basic understanding, you’ll be able to create useful macros and automate your workflow.
Final Thoughts
Learning VBA can save you hours of time and give you a new level of control over Excel and other Office programs. It’s not as hard as it looks, especially if you start small. So, why not give it a try? Write your first macro, play around with variables and loops, and soon enough, you’ll be automating your tasks like a pro!