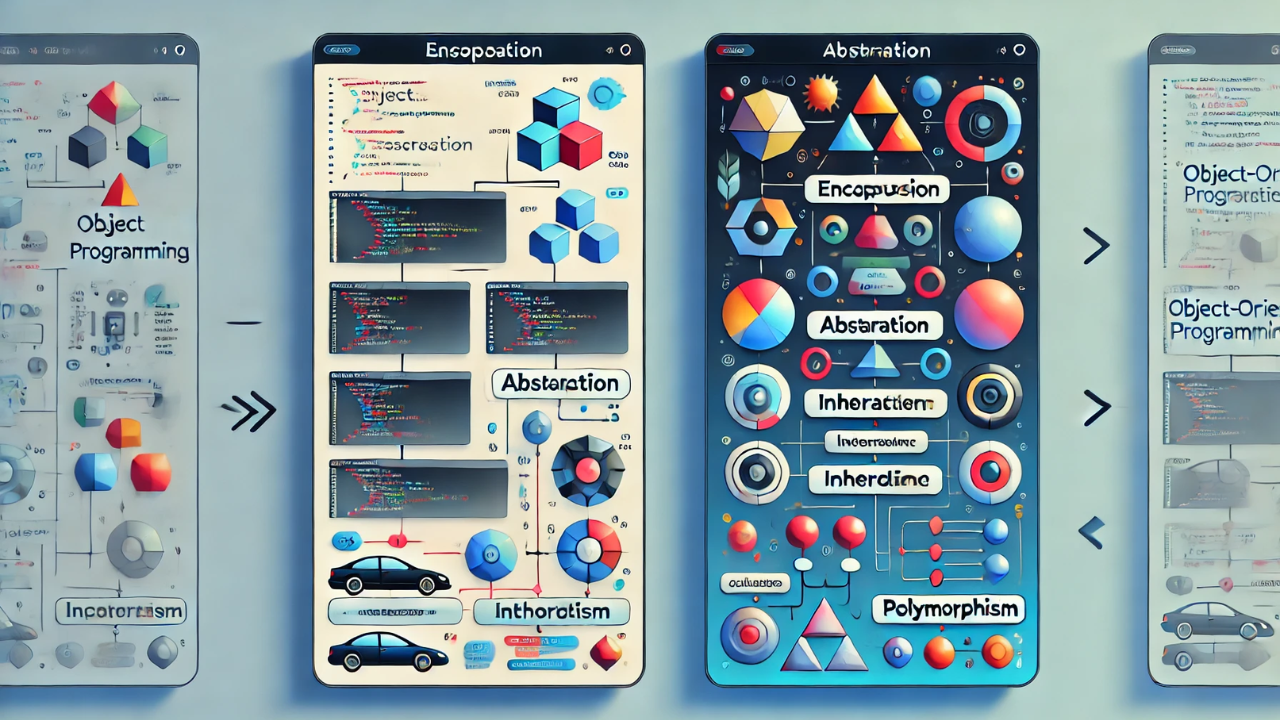
Object-Oriented Programming (OOP) is a fundamental concept in modern software development. It’s a paradigm that helps developers create more organized, modular, and maintainable code. In this guide, we’ll delve into the basics of OOP in C++, exploring its core principles and demonstrating how you can leverage them in your programming projects.
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of “objects.” Objects are instances of classes, which can contain both data (attributes) and functions (methods). This approach allows for better organization, reuse, and abstraction of code.
Key Principles of OOP
OOP in C++ is built on four main principles:
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
Let’s break each one down.
1. Encapsulation
Encapsulation involves bundling data and methods that operate on that data within a single unit or class. It restricts direct access to some of the object’s components, which is a means of preventing accidental interference and misuse.
Example:
class Car {
private:
int speed;
public:
void setSpeed(int s) {
speed = s;
}
int getSpeed() {
return speed;
}
};
In the above example, speed
is a private attribute, meaning it can’t be accessed directly from outside the Car
class. The setSpeed
and getSpeed
methods provide controlled access.
2. Abstraction
Abstraction is about hiding the complex implementation details and showing only the essential features of the object. It helps in reducing programming complexity and effort.
Example:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override {
cout << "Woof!" << endl;
}
};
class Cat : public Animal {
public:
void sound() override {
cout << "Meow!" << endl;
}
};
In this example, Animal
is an abstract class with a pure virtual function sound()
. The Dog
and Cat
classes provide concrete implementations of this function.
3. Inheritance
Inheritance allows a new class to inherit attributes and methods from an existing class. This promotes code reuse and establishes a natural hierarchy between classes.
Example:
class Vehicle {
public:
int wheels;
void setWheels(int w) {
wheels = w;
}
int getWheels() {
return wheels;
}
};
class Bike : public Vehicle {
public:
void showBike() {
cout << "Bike with " << wheels << " wheels" << endl;
}
};
Here, the Bike
class inherits from the Vehicle
class, meaning it can use the setWheels
and getWheels
methods.
4. Polymorphism
Polymorphism means “many forms” and it allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation.
Example:
class Shape {
public:
virtual void draw() {
cout << "Drawing a shape" << endl;
}
};
class Circle : public Shape {
public:
void draw() override {
cout << "Drawing a circle" << endl;
}
};
class Square : public Shape {
public:
void draw() override {
cout << "Drawing a square" << endl;
}
};
void drawShape(Shape &s) {
s.draw();
}
In this example, the draw
function is overridden in the Circle
and Square
classes. The drawShape
function can call draw
on any Shape
object, demonstrating polymorphism.
Benefits of OOP in C++
Using OOP in C++ offers several advantages:
- Modularity: Code is organized into discrete classes, making it easier to manage and understand.
- Reusability: Classes can be reused across different programs.
- Scalability: Programs can grow more complex without becoming unmanageable.
- Maintainability: Code is easier to maintain and update.
it’s here Games Programmed with C++ and Java.
Practical Example: Building a Simple Bank Account System
Let’s apply what we’ve learned to create a simple bank account system in C++.
#include <iostream>
using namespace std;
class BankAccount {
private:
string owner;
double balance;
public:
BankAccount(string o, double b) : owner(o), balance(b) {}
void deposit(double amount) {
balance += amount;
cout << "Deposited: " << amount << ", New Balance: " << balance << endl;
}
void withdraw(double amount) {
if (amount > balance) {
cout << "Insufficient funds!" << endl;
} else {
balance -= amount;
cout << "Withdrawn: " << amount << ", New Balance: " << balance << endl;
}
}
void display() {
cout << "Owner: " << owner << ", Balance: " << balance << endl;
}
};
int main() {
BankAccount account("Alice", 1000.0);
account.display();
account.deposit(200.0);
account.withdraw(500.0);
account.withdraw(800.0);
return 0;
}
FAQs
What is OOP in C++?
OOP in C++ is a programming paradigm that uses “objects” to design software. It includes principles like encapsulation, abstraction, inheritance, and polymorphism.
Why use OOP?
OOP helps in organizing complex code, promoting reuse, and making software easier to manage and maintain.
Can you give a simple example of OOP in C++?
Sure, the BankAccount
class example above demonstrates encapsulation, with methods to deposit, withdraw, and display account information.
Final Thoughts
Understanding and applying OOP principles in C++ can significantly enhance your programming skills. By organizing code into classes and objects, you can create more robust, maintainable, and scalable applications. So, roll up your sleeves, dive into C++, and start exploring the world of Object-Oriented Programming!