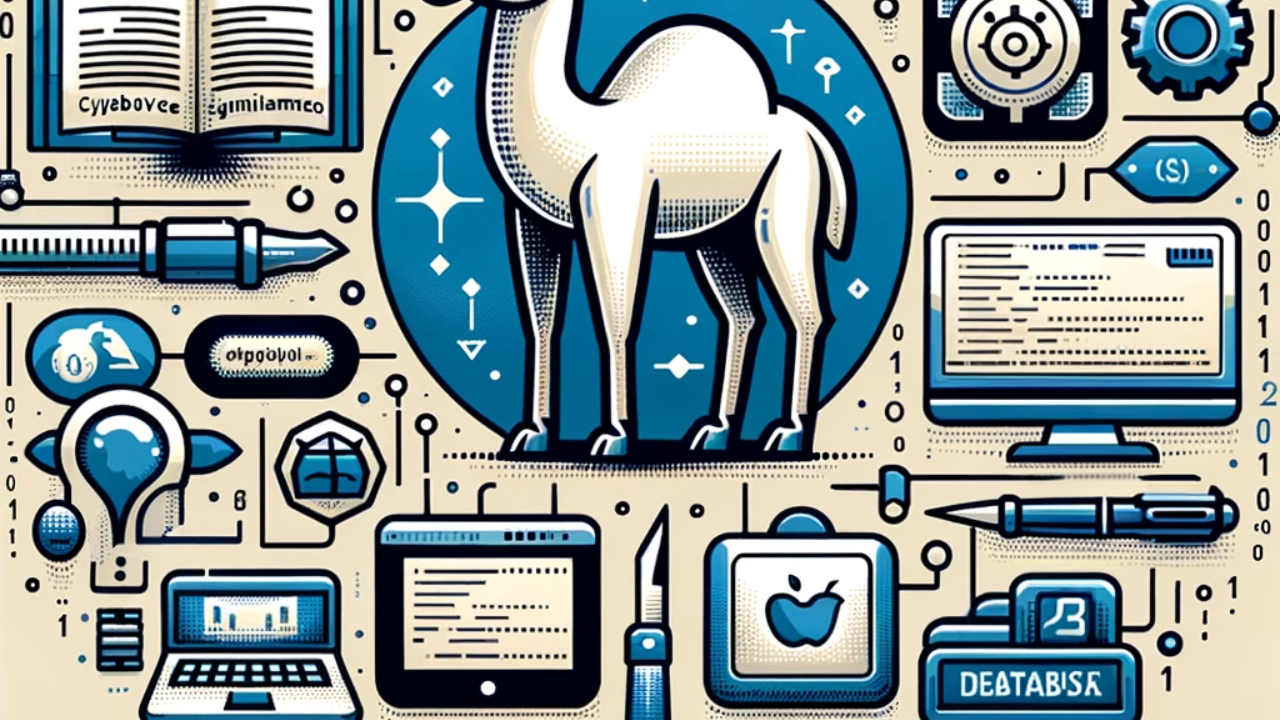
Perl, often dubbed the “Swiss Army knife” of programming languages, is known for its flexibility and power in handling text and data. Created by Larry Wall in 1987, Perl has evolved over the years and remains a go-to language for system administration, web development, and more. Let’s explore Perl in detail and see how it can be a valuable tool in your programming arsenal.
What is Perl?
Perl stands for “Practical Extraction and Report Language.” It’s a high-level, interpreted language renowned for its capability in text manipulation. With a rich set of features and an active community, Perl is particularly popular for:
- Text Processing: Perl’s strong support for regular expressions makes it perfect for text and string manipulation.
- System Administration: Its ability to interact with the system makes it a favorite among sysadmins.
- Web Development: Many web applications and CGI scripts are written in Perl.
- Database Interaction: Perl can connect to various databases, making it suitable for backend programming.
Key Features of Perl
- Flexibility and Versatility:
Perl allows multiple ways to accomplish a task. This flexibility enables programmers to choose the most suitable method for their specific needs. - Powerful Text Processing:
With its robust regular expression engine, Perl excels in tasks involving text manipulation, data extraction, and reporting. - Cross-Platform Compatibility:
Perl runs on almost any platform, including Unix, Linux, Windows, and macOS, providing great portability for your scripts. - Comprehensive Library (CPAN):
The Comprehensive Perl Archive Network (CPAN) is a vast repository of Perl modules and extensions, allowing you to reuse code and expand Perl’s functionality. - Community Support:
Perl boasts a large and active community, offering extensive documentation, forums, and mailing lists for support.
Rust Programming Language you need to know
Getting Started with Perl
Installation
To start using Perl, you need to install it on your system. Most Unix-like systems come with Perl pre-installed. For Windows, you can use Strawberry Perl or ActivePerl.
Writing Your First Perl Script
Let’s write a simple “Hello, World!” program in Perl.
#!/usr/bin/perl
print "Hello, World!\n";
Save this file with a .pl
extension, for example, hello.pl
. To run it, open your terminal or command prompt and type:
perl hello.pl
You should see the output:
Hello, World!
Perl Syntax and Basics
Variables
Perl uses three main types of variables:
- Scalars: Represent single values (numbers, strings, etc.)
my $name = "John";
my $age = 30;
- Arrays: Ordered lists of scalars
my @colors = ("red", "green", "blue");
- Hashes: Unordered sets of key-value pairs
my %fruit_colors = ("apple" => "red", "banana" => "yellow");
Control Structures
Perl supports typical control structures like if
, unless
, while
, and for
.
my $number = 10;
if ($number > 5) {
print "The number is greater than 5\n";
} else {
print "The number is 5 or less\n";
}
Subroutines
Subroutines in Perl are used to encapsulate code for reuse.
sub greet {
my ($name) = @_;
print "Hello, $name!\n";
}
greet("Alice");
Advanced Perl Concepts
Regular Expressions
Perl’s regular expressions are incredibly powerful for pattern matching and text manipulation.
my $text = "The quick brown fox jumps over the lazy dog.";
if ($text =~ /quick/) {
print "Found 'quick' in the text.\n";
}
File Handling
Reading from and writing to files is straightforward in Perl.
# Reading from a file
open(my $fh, '<', 'input.txt') or die "Cannot open input.txt: $!";
while (my $line = <$fh>) {
print $line;
}
close($fh);
# Writing to a file
open(my $fh, '>', 'output.txt') or die "Cannot open output.txt: $!";
print $fh "Hello, World!\n";
close($fh);
Real-World Examples
Web Scraping
Using Perl’s LWP module, you can easily scrape web content.
use LWP::Simple;
my $content = get('http://example.com') or die 'Could not get content';
print $content;
Database Interaction
With the DBI module, Perl can interact with databases seamlessly.
use DBI;
my $dbh = DBI->connect("DBI:mysql:database=testdb;host=localhost", "user", "password");
my $sth = $dbh->prepare("SELECT * FROM users");
$sth->execute();
while (my @row = $sth->fetchrow_array) {
print "User: $row[0]\n";
}
$sth->finish;
$dbh->disconnect;
Apple’s Programming Language (swift) you need to know
Conclusion
Perl is a powerful, flexible language that excels in text processing, system administration, and more. With its extensive libraries and strong community support, Perl remains a valuable tool for many programming tasks. Whether you’re a beginner or an experienced programmer, learning Perl can open up a world of possibilities.
FAQs
Q: What is Perl mainly used for?
A: Perl is primarily used for text processing, system administration, web development, and database interaction.
Q: Is Perl easy to learn?
A: Perl’s syntax is relatively straightforward, and its extensive documentation and community support make it accessible for beginners.
Q: How does Perl handle regular expressions?
A: Perl has a built-in, powerful regular expression engine, making it ideal for tasks involving pattern matching and text manipulation.