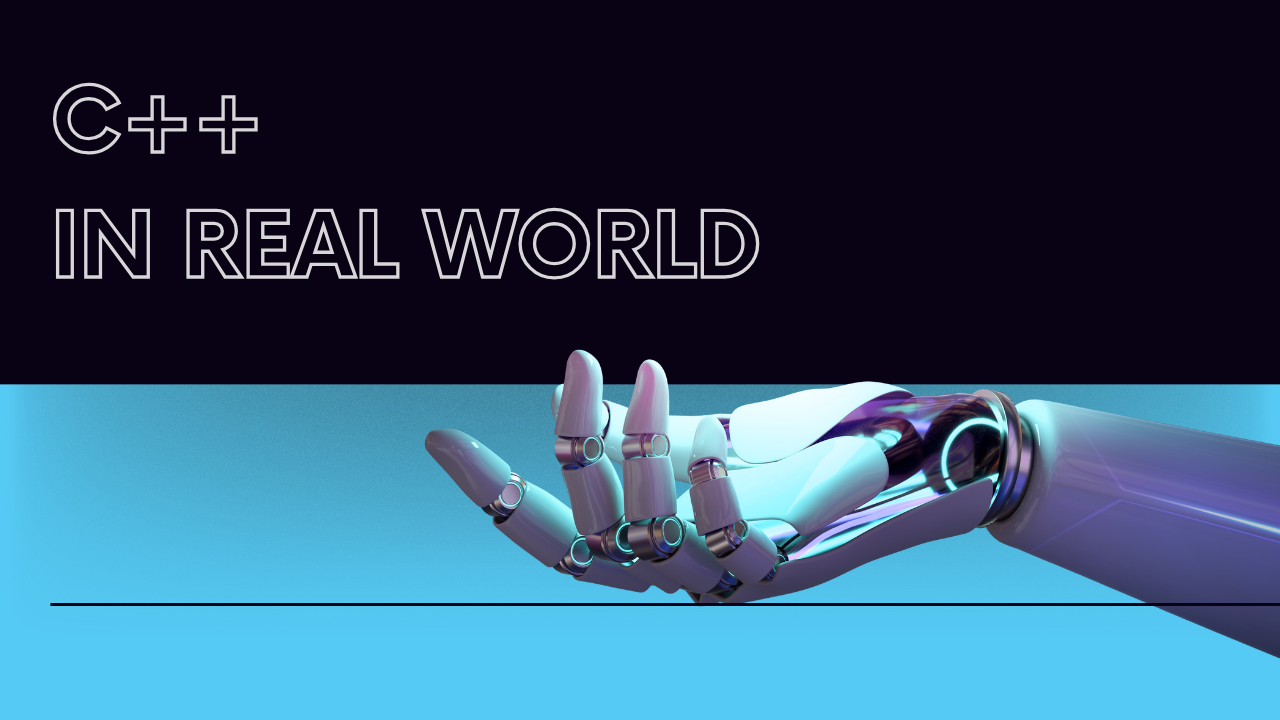
C++ is a powerful programming language that’s been around for decades, helping build everything from video games to financial systems. But how exactly is it used in the real world? Let’s dive into some practical applications and simple examples to understand its impact better.
Gaming Development
C++ is a cornerstone in the gaming industry. Its performance and control over system resources make it perfect for game development. Big titles like “World of Warcraft” and “Counter-Strike” are built using C++.
Example: Basic Game Loop
Here’s a simplified example of a basic game loop in C++:
#include <iostream>
using namespace std;
bool isRunning = true;
void gameLoop() {
while (isRunning) {
cout << "Game is running..." << endl;
// Game logic goes here
// For demonstration, we'll stop the loop
isRunning = false;
}
}
int main() {
gameLoop();
cout << "Game has ended." << endl;
return 0;
}
This snippet keeps the game running until we decide to stop it, illustrating how fundamental game loops are in C++.
It’s here Data Structures in C++ need to know.
Systems Programming
C++ is used extensively for systems programming, which involves building operating systems and hardware drivers. Its ability to interact closely with hardware makes it ideal for this purpose.
Example: Basic File Handling
File handling is crucial in systems programming. Here’s how you can read and write to a file in C++:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream myfile("example.txt");
myfile << "Writing this to a file.\n";
myfile.close();
ifstream myReadFile("example.txt");
string line;
if (myReadFile.is_open()) {
while (getline(myReadFile, line)) {
cout << line << endl;
}
myReadFile.close();
}
return 0;
}
This code demonstrates writing to a file and then reading from it, showcasing C++’s efficiency in handling file operations.
Financial Systems
Banks and financial institutions use C++ for its speed and reliability. High-frequency trading systems and risk management applications often rely on C++.
Example: Basic Interest Calculation
Let’s calculate simple interest using C++:
#include <iostream>
using namespace std;
int main() {
double principal, rate, time, interest;
cout << "Enter principal amount: ";
cin >> principal;
cout << "Enter rate of interest: ";
cin >> rate;
cout << "Enter time period in years: ";
cin >> time;
interest = (principal * rate * time) / 100;
cout << "Simple Interest is: " << interest << endl;
return 0;
}
This straightforward program calculates interest based on user inputs, highlighting C++’s practicality in financial calculations.
Ultimate Power of Pointers in C you have to know
Web Browsers
Browsers like Chrome and Firefox use C++ to handle performance-critical tasks. Its efficiency ensures faster processing and a smoother user experience.
Example: Basic URL Validator
Here’s a simple C++ program to validate a URL format:
#include <iostream>
#include <regex>
using namespace std;
bool isValidURL(const string& url) {
const regex pattern("(http|https)://(\\w+)(\\.\\w+)+");
return regex_match(url, pattern);
}
int main() {
string url;
cout << "Enter a URL: ";
cin >> url;
if (isValidURL(url)) {
cout << "Valid URL" << endl;
} else {
cout << "Invalid URL" << endl;
}
return 0;
}
This code checks if the entered URL matches a basic pattern, demonstrating C++’s capability in web-related tasks.
Embedded Systems
C++ is widely used in embedded systems, which are specialized computing systems that perform dedicated functions. From microwave ovens to medical devices, C++ helps run these critical systems efficiently.
Example: Basic LED Control
Imagine a simple program to control an LED on an embedded system:
#include <iostream>
using namespace std;
void turnOnLED() {
cout << "LED is ON" << endl;
}
void turnOffLED() {
cout << "LED is OFF" << endl;
}
int main() {
turnOnLED();
// Assume some condition or delay
turnOffLED();
return 0;
}
This program illustrates the basic control flow for turning an LED on and off, a common task in embedded programming.
Different b/w C and C++ increase your skill
Conclusion
C++ is everywhere, from the games we play to the systems that power our daily lives. Its versatility and performance make it a favorite among developers. By understanding these real-world examples, you can appreciate the significant role C++ plays in technology today.