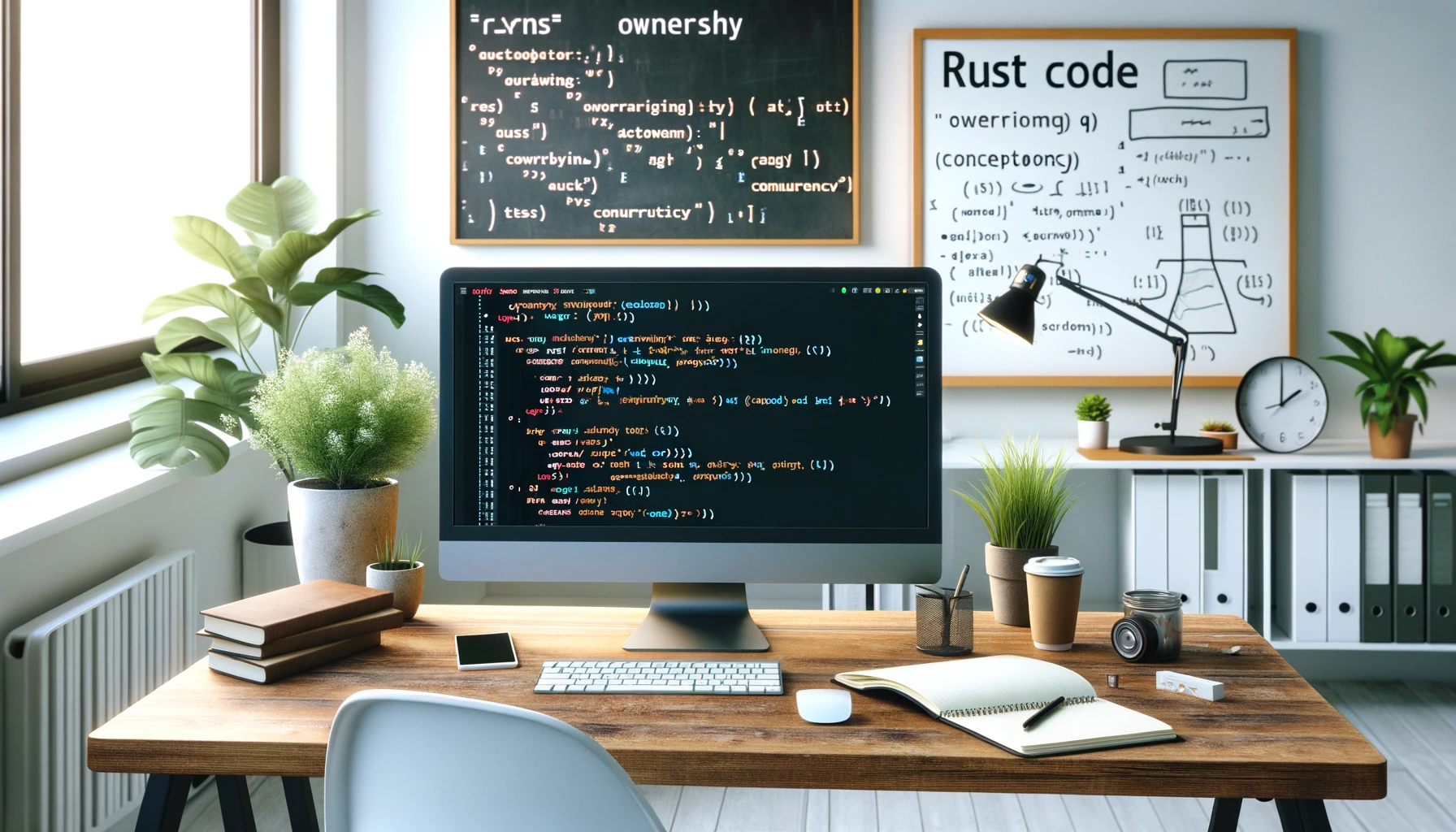
Rust is quickly becoming a favorite among developers, and for good reason. It’s designed for performance and safety, making it ideal for systems programming. If you’re curious about Rust and want to learn more, you’ve come to the right place!
In this blog, we’ll break down what makes Rust special, its key features, and how you can start coding in Rust with simple examples.
What Makes Rust Special?
Rust is a systems programming language that’s been gaining traction for its ability to provide memory safety without using a garbage collector. This means you get the speed and efficiency of languages like C and C++ without the usual pitfalls like memory leaks and data races.
Key Features of Rust
- Memory Safety: Rust’s ownership system ensures memory safety and prevents data races.
- Concurrency: Built-in support for concurrent programming makes it easier to write multi-threaded applications.
- Performance: Rust is fast and efficient, suitable for high-performance applications.
- Reliability: The compiler checks for a range of errors, ensuring your code is more reliable.
- Community and Ecosystem: A growing community and rich ecosystem of libraries and tools.
Apple’s Programming Language (swift) you need to know
Getting Started with Rust
First things first, you’ll need to install Rust. Head over to rust-lang.org and follow the instructions for your operating system.
Once installed, you can start writing Rust code. Let’s dive into some examples.
Hello, World!
Let’s start with the classic “Hello, World!” program.
fn main() {
println!("Hello, World!");
}
This simple program defines a main
function and uses the println!
macro to print text to the console.
Variables and Mutability
In Rust, variables are immutable by default. If you want a mutable variable, you need to use the mut
keyword.
fn main() {
let x = 5; // Immutable
println!("The value of x is: {}", x);
let mut y = 10; // Mutable
println!("The value of y is: {}", y);
y = 15;
println!("The new value of y is: {}", y);
}
Control Flow
Rust supports typical control flow structures like if
, else
, and loops.
If-Else
fn main() {
let number = 6;
if number % 2 == 0 {
println!("The number is even.");
} else {
println!("The number is odd.");
}
}
Loops
Rust has several kinds of loops, including loop
, while
, and for
.
fn main() {
let mut count = 0;
// Infinite loop
loop {
count += 1;
if count == 3 {
break;
}
println!("Count is: {}", count);
}
// While loop
while count < 5 {
count += 1;
println!("Count in while loop: {}", count);
}
// For loop
for i in 1..5 {
println!("Count in for loop: {}", i);
}
}
Functions
Functions in Rust are simple to define and use.
fn main() {
greet("Alice");
let sum = add(5, 3);
println!("The sum is: {}", sum);
}
fn greet(name: &str) {
println!("Hello, {}!", name);
}
fn add(a: i32, b: i32) -> i32 {
a + b
}
Ownership and Borrowing
One of Rust’s standout features is its ownership system, which helps manage memory.
Ownership
fn main() {
let s1 = String::from("hello");
let s2 = s1;
// println!("{}", s1); // This would cause a compile-time error because s1 is moved to s2
println!("{}", s2);
}
Borrowing
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
}
Structs and Enums
Rust allows you to define custom types using structs and enums.
Structs
struct User {
username: String,
email: String,
active: bool,
}
fn main() {
let user1 = User {
username: String::from("john_doe"),
email: String::from("john@example.com"),
active: true,
};
println!("Username: {}", user1.username);
}
Enums
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
ChangeColor(i32, i32, i32),
}
fn main() {
let msg1 = Message::Write(String::from("Hello, Rust!"));
match msg1 {
Message::Quit => println!("Quit message"),
Message::Move { x, y } => println!("Move to ({}, {})", x, y),
Message::Write(text) => println!("Text message: {}", text),
Message::ChangeColor(r, g, b) => println!("Change color to RGB({}, {}, {})", r, g, b),
}
}
Error Handling
Rust provides robust error handling with Result
and Option
types.
Result
use std::fs::File;
use std::io::ErrorKind;
fn main() {
let file = File::open("hello.txt");
let _file = match file {
Ok(file) => file,
Err(ref error) if error.kind() == ErrorKind::NotFound => {
match File::create("hello.txt") {
Ok(fc) => fc,
Err(e) => panic!("Problem creating the file: {:?}", e),
}
}
Err(error) => {
panic!("Problem opening the file: {:?}", error)
}
};
}
Option
fn main() {
let some_number = Some(5);
let absent_number: Option<i32> = None;
println!("Some number: {:?}", some_number);
println!("Absent number: {:?}", absent_number);
}
Conclusion
Rust is a powerful, fast, and safe programming language. Its unique features like ownership and borrowing make it stand out, especially for systems programming. Whether you’re building a small utility or a large system, Rust provides the tools and safety guarantees to get the job done right.
So, why not give Rust a try? Install it, write some code, and see for yourself why so many developers are making the switch to Rust.
It’s here PIP Python Package Manager you need to know
FAQs
Q: What makes Rust different from other programming languages?
A: Rust’s ownership system ensures memory safety and prevents data races without a garbage collector, which makes it unique compared to other languages.
Q: Is Rust suitable for beginners?
A: Yes, Rust has a steep learning curve, but its comprehensive documentation and helpful community make it accessible for beginners.
Q: Can Rust be used for web development?
A: Absolutely! With frameworks like Rocket and Actix, Rust is becoming a popular choice for web development.
Q: How does Rust handle concurrency?
A: Rust has built-in support for concurrent programming, making it easier to write safe and efficient multi-threaded applications.