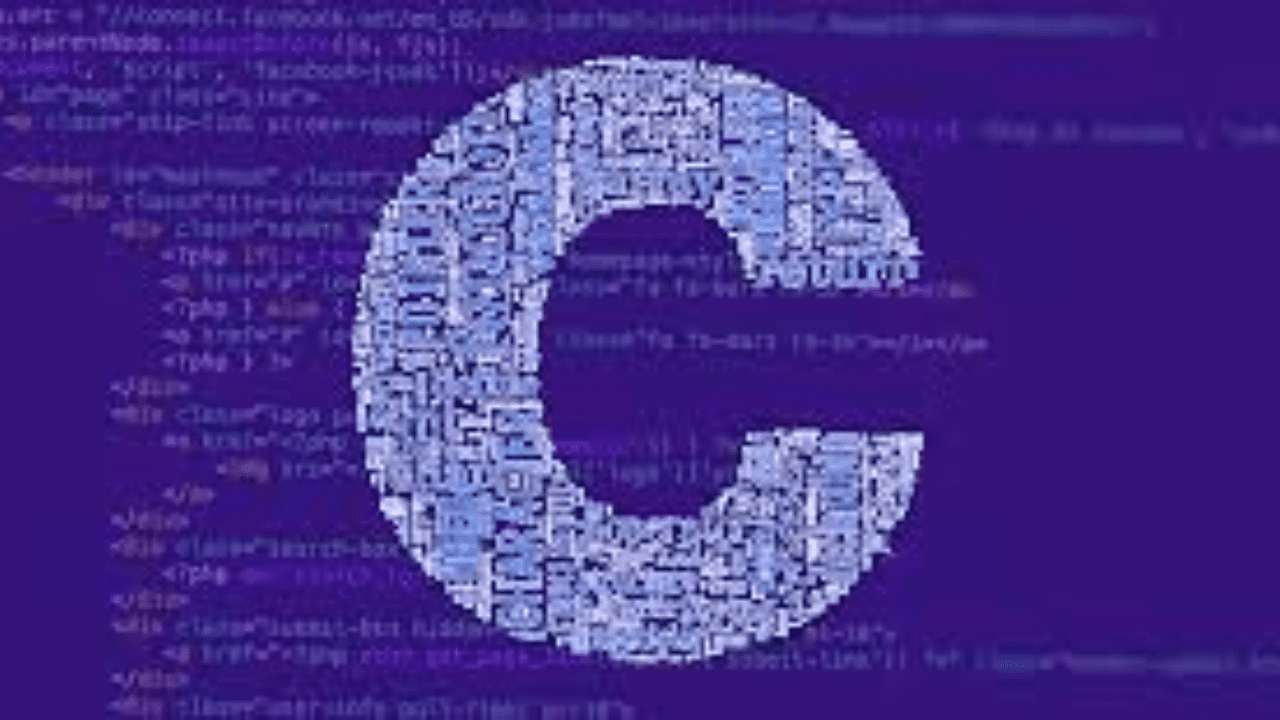
A pointer in C is a variable that stores the memory address of another variable.
It allows direct manipulation of memory, facilitating dynamic memory allocation and efficient access to data structures.
Syntax of C Pointers:
data_type *pointer_name;
– `data_type`: The type of data that the pointer points to.
– `pointer_name`: The name of the pointer.
How to Use Pointers:
Pointer Declaration:
int *ptr; // Declaring a pointer to an integer
Pointer Initialization:
int x = 10;
int *ptr = &x; // Initializing pointer with the address of variable x
Pointer Dereferencing:
int y = *ptr; // Dereferencing pointer to get the value at the address it points to
Types of Pointers in C:
Null Pointer:
int *ptr = NULL;
Void Pointer:
void *generic_ptr;
Pointer to Pointer (Double Pointer):
int x = 10;
int *ptr1 = &x;
int ptr2 = &ptr1;
Other Types of Pointers in C:
Function Pointers:
int (*func_ptr)(int, int);
Array Pointers:
int arr[5];
int *arr_ptr = arr;
How to find the size of pointers in C?
You can use the `sizeof` operator:
printf("Size of pointer: %lu bytes\n", sizeof(int *));
C Pointer Arithmetic:
Pointer arithmetic involves adding or subtracting an integer to/from a pointer. It is scaled based on the size of the data type.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = arr;
// Incrementing pointer
ptr++;
// Decrementing pointer
ptr--;
// Accessing array elements using pointer arithmetic
int thirdElement = *(ptr + 2);
C Pointers and Arrays:
Arrays and pointers are closely related in C, and array names are essentially pointers to the first element of the array.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = arr; // Equivalent to int *ptr = &arr[0];
Uses of Pointers in C:
Dynamic Memory Allocation:
int *arr = (int *)malloc(5 * sizeof(int));
Passing Parameters to Functions:
void modifyValue(int *x) {
(*x)++;
}
3. Manipulating Arrays and Strings:
char str[] = "Hello";
char *ptr = str;
Advantages of Pointers:
Dynamic Memory Allocation:
Allows efficient memory management.
Efficient Parameter Passing:
Reduces the overhead of passing large data structures.
Efficient Access to Data Structures:
Enables easy traversal and manipulation of complex data structures.
Disadvantages of Pointers:
Dangling Pointers:
Pointers may become invalid if they point to deallocated memory.
Memory Leaks:
Failure to free allocated memory can lead to memory leaks.
Complexity:
Pointers add complexity and can lead to bugs if not used carefully.
comment for more coding questions!
Different b/w C and C++ increase your skill
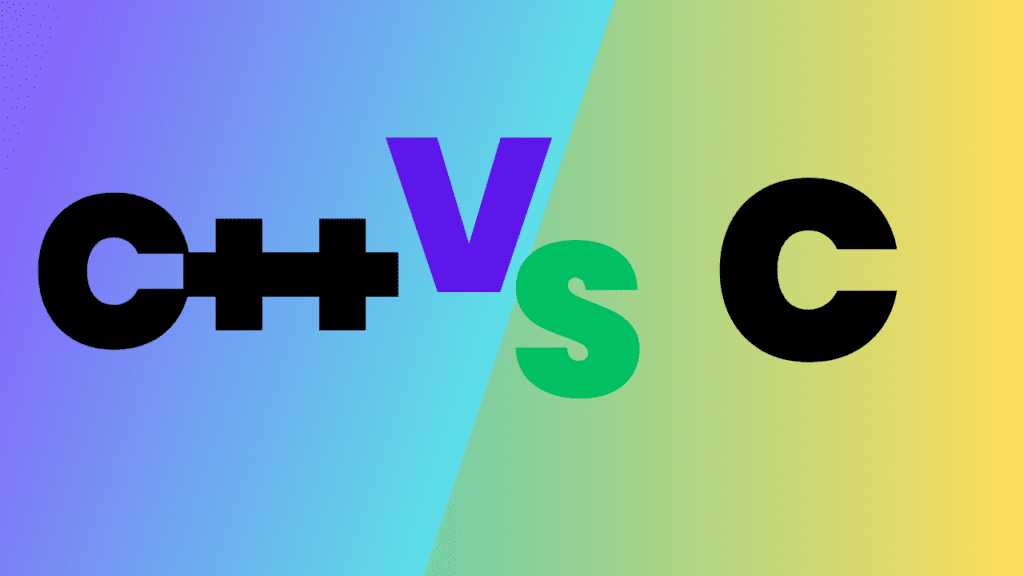
Popular sorting techniques and you don’t know.
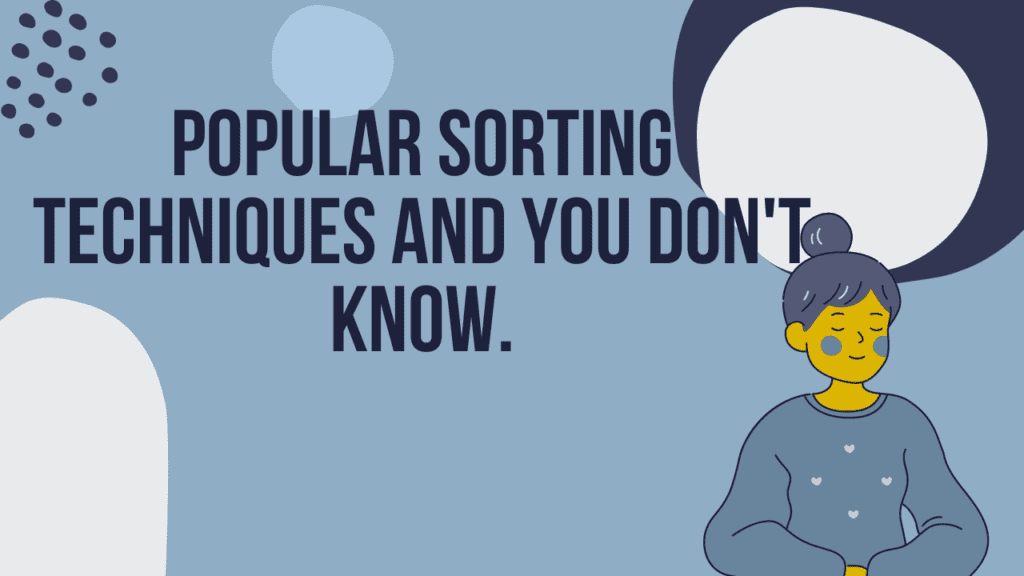