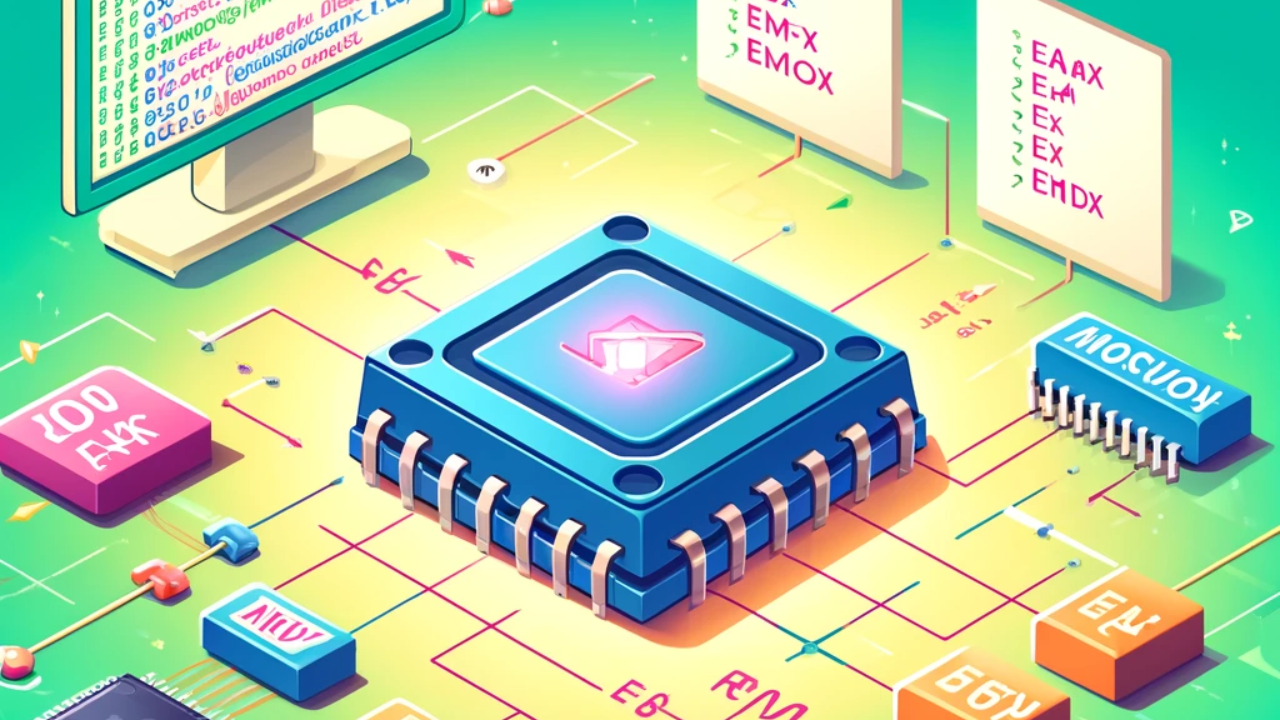
Assembly language, a low-level programming language, is often considered daunting. But don’t worry – we’re here to make it simple and approachable. This blog will cover the basics, show you some real-life examples, and explain why learning assembly language can be incredibly beneficial.
What is Assembly Language?
Assembly language is a type of low-level programming language that is closely related to machine code, the fundamental language of computers. It acts as a bridge between high-level languages (like Python or Java) and the machine code that a computer’s processor understands.
Each type of processor has its own assembly language. This is because assembly language directly corresponds to the machine instructions that the processor executes.
Why Learn Assembly Language?
- Understanding How Computers Work: Learning assembly language helps you understand how software interacts with hardware.
- Efficiency: Programs written in assembly language can be more efficient than those written in higher-level languages because they allow for precise control over the computer’s resources.
- Embedded Systems: Many embedded systems and critical applications are written in assembly language due to its efficiency and control.
Rust Programming Language you need to know
Basics of Assembly Language
Let’s start with some fundamental concepts:
- Instructions: Assembly language consists of instructions that tell the CPU what to do.
- Registers: Small storage locations within the CPU used to perform operations.
- Operands: The data that the instructions operate on.
- Mnemonics: Human-readable representations of machine code instructions (e.g.,
MOV
,ADD
).
Here’s a simple example of assembly language for the x86 architecture:
section .data
msg db 'Hello, World!', 0 ; Define a message
section .text
global _start ; Entry point for the program
_start:
; Write message to stdout
mov eax, 4 ; syscall number for sys_write
mov ebx, 1 ; file descriptor 1 (stdout)
mov ecx, msg ; pointer to the message
mov edx, 13 ; length of the message
int 0x80 ; make the system call
; Exit the program
mov eax, 1 ; syscall number for sys_exit
xor ebx, ebx ; exit code 0
int 0x80 ; make the system call
Breaking Down the Example
- Data Section: The
section .data
declares initialized data or constants. Here,msg db 'Hello, World!', 0
stores the string “Hello, World!” followed by a null terminator (0
). - Text Section: The
section .text
contains the code. - Global _start: The
_start
label is the entry point of the program. - System Call for Writing to stdout:
mov eax, 4
: Move the value4
(the syscall number forsys_write
) into theeax
register.mov ebx, 1
: Move the value1
(the file descriptor for stdout) into theebx
register.mov ecx, msg
: Move the address ofmsg
into theecx
register.mov edx, 13
: Move the length of the message (13
characters) into theedx
register.int 0x80
: Make the system call.
- System Call for Exiting:
mov eax, 1
: Move the value1
(the syscall number forsys_exit
) into theeax
register.xor ebx, ebx
: Zero out theebx
register to set the exit code to0
.int 0x80
: Make the system call.
More Assembly Language Concepts
- Loops: Repeating a set of instructions.
- Conditionals: Making decisions based on certain conditions.
- Functions: Grouping instructions into reusable blocks.
Here’s a quick example of a loop in assembly language:
section .bss
count resb 1 ; Reserve 1 byte for count
section .text
global _start
_start:
mov byte [count], 10 ; Initialize count to 10
loop_start:
dec byte [count] ; Decrement count
cmp byte [count], 0 ; Compare count with 0
jne loop_start ; Jump to loop_start if count is not zero
; Exit the program
mov eax, 1
xor ebx, ebx
int 0x80
In this example:
- Data Section (
.bss
): Reserves 1 byte forcount
. - Initialize Count:
mov byte [count], 10
setscount
to 10. - Loop:
dec byte [count]
: Decrementscount
.cmp byte [count], 0
: Comparescount
with 0.jne loop_start
: Jumps back toloop_start
ifcount
is not zero.
FAQs About Assembly Language
Q: Is assembly language hard to learn?
A: It can be challenging at first, but with practice and understanding of how computers work, it becomes easier.
Q: What are registers?
A: Registers are small storage locations within the CPU used to perform operations quickly.
Q: Why is assembly language still used?
A: It’s used for efficiency and control, especially in embedded systems and performance-critical applications.
Apple’s Programming Language (swift) you need to know
Wrapping Up
Understanding assembly language gives you a deeper insight into how computers operate. It may seem complex, but breaking it down into simple instructions, as we’ve done here, can make it much more approachable. With practice, you’ll be able to write efficient, low-level code and better appreciate the inner workings of your machine.
Feel free to ask any questions or leave comments below. Happy coding!