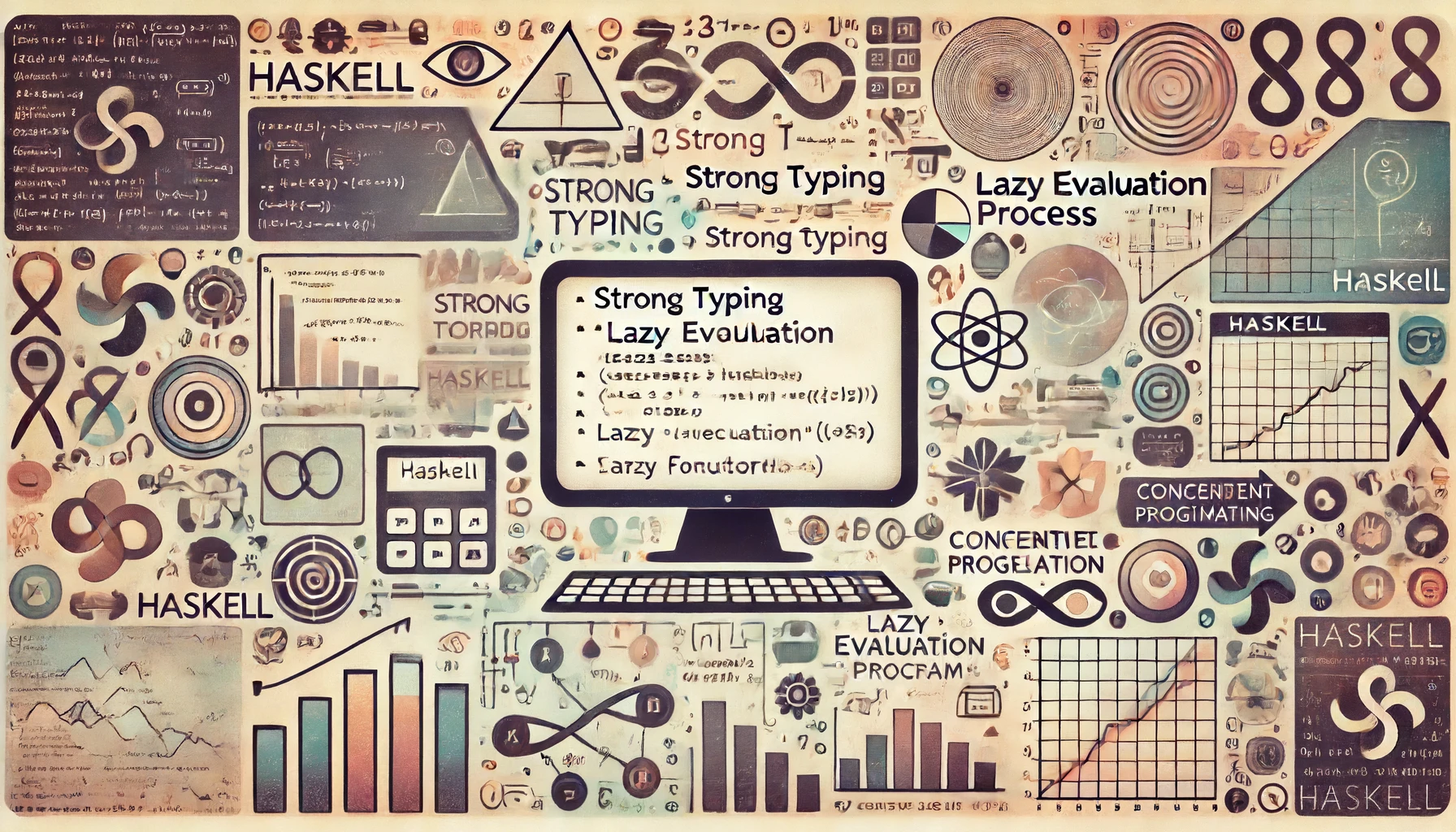
Haskell is a functional programming language that’s known for its expressive syntax, powerful type system, and lazy evaluation. Whether you’re new to programming or looking to expand your skills, Haskell offers a unique approach that can enhance your coding capabilities. In this guide, we’ll explore the basics of Haskell, highlight its key features, and walk through some examples to help you get started.
What is Haskell?
Haskell is a purely functional programming language named after the mathematician Haskell Curry. Unlike imperative languages like Python or Java, Haskell focuses on what to solve rather than how to solve it. This makes Haskell code concise, readable, and often more robust.
Why Learn Haskell?
- Functional Paradigm: Emphasizes functions and their applications.
- Strong Typing: Reduces runtime errors.
- Lazy Evaluation: Only computes what is necessary.
- Conciseness: Express complex ideas with less code.
Getting Started with Haskell
Before diving into examples, you need to set up Haskell on your machine. You can install the Haskell Platform, which includes the GHC compiler and useful libraries. Visit the Haskell website for installation instructions.
Coding for Kids with Scratch you need to know
Basic Syntax and Concepts
Let’s start with some fundamental concepts and syntax in Haskell.
Hello, World!
main :: IO ()
main = putStrLn "Hello, World!"
This simple program prints “Hello, World!” to the console. Here’s a breakdown:
main :: IO ()
specifies the type ofmain
, indicating it performs input/output operations.putStrLn "Hello, World!"
is a function that outputs the string to the console.
Functions
Functions are the core of Haskell. Here’s how you define and use them:
-- Define a function
add :: Int -> Int -> Int
add x y = x + y
-- Use the function
main :: IO ()
main = print (add 2 3)
In this example:
add :: Int -> Int -> Int
defines the type ofadd
which takes two integers and returns an integer.add x y = x + y
defines the function itself.print (add 2 3)
calls the function and prints the result.
Lists
Lists are a fundamental data structure in Haskell:
-- Creating a list
numbers :: [Int]
numbers = [1, 2, 3, 4, 5]
-- List operations
sumNumbers :: Int
sumNumbers = sum numbers
numbers :: [Int]
defines a list of integers.sumNumbers = sum numbers
calculates the sum of the list.
Pattern Matching
Pattern matching is a powerful feature in Haskell that simplifies code involving different cases:
-- Define a function using pattern matching
factorial :: Int -> Int
factorial 0 = 1
factorial n = n * factorial (n - 1)
This recursive function calculates the factorial of a number.
Advanced Concepts
Once you’re comfortable with the basics, you can explore more advanced topics like:
Higher-Order Functions
Functions that take other functions as arguments:
-- Higher-order function example
applyTwice :: (a -> a) -> a -> a
applyTwice f x = f (f x)
Here, applyTwice
takes a function f
and applies it twice to x
.
Lazy Evaluation
Haskell uses lazy evaluation, meaning it delays computations until absolutely necessary:
-- Infinite list example
numbers :: [Int]
numbers = [1..]
-- Take the first 5 numbers
firstFive :: [Int]
firstFive = take 5 numbers
Even though numbers
is an infinite list, take 5 numbers
only evaluates the first five elements.
Monads
Monads handle side effects and represent computations instead of values:
-- Maybe Monad example
safeDivide :: Int -> Int -> Maybe Int
safeDivide _ 0 = Nothing
safeDivide x y = Just (x `div` y)
safeDivide
returns Nothing
if there’s an attempt to divide by zero, otherwise it returns the division result wrapped in Just
.
Its here Mastering React Programming you need to know.
Conclusion
Haskell is a powerful language that can change the way you think about programming. Its emphasis on functional programming, strong typing, and lazy evaluation makes it unique and rewarding to learn. Start with the basics, experiment with functions and lists, and soon you’ll appreciate the elegance and power of Haskell.
FAQs
Q: What are the benefits of using Haskell?
A: Haskell offers concise and readable code, strong typing, and lazy evaluation which can lead to more efficient programs.
Q: Is Haskell difficult to learn?
A: Haskell can be challenging initially, especially if you’re used to imperative languages, but with practice, its concepts become clear and intuitive.
Q: What can I build with Haskell?
A: Haskell is used in various fields including web development, data analysis, and concurrent programming.