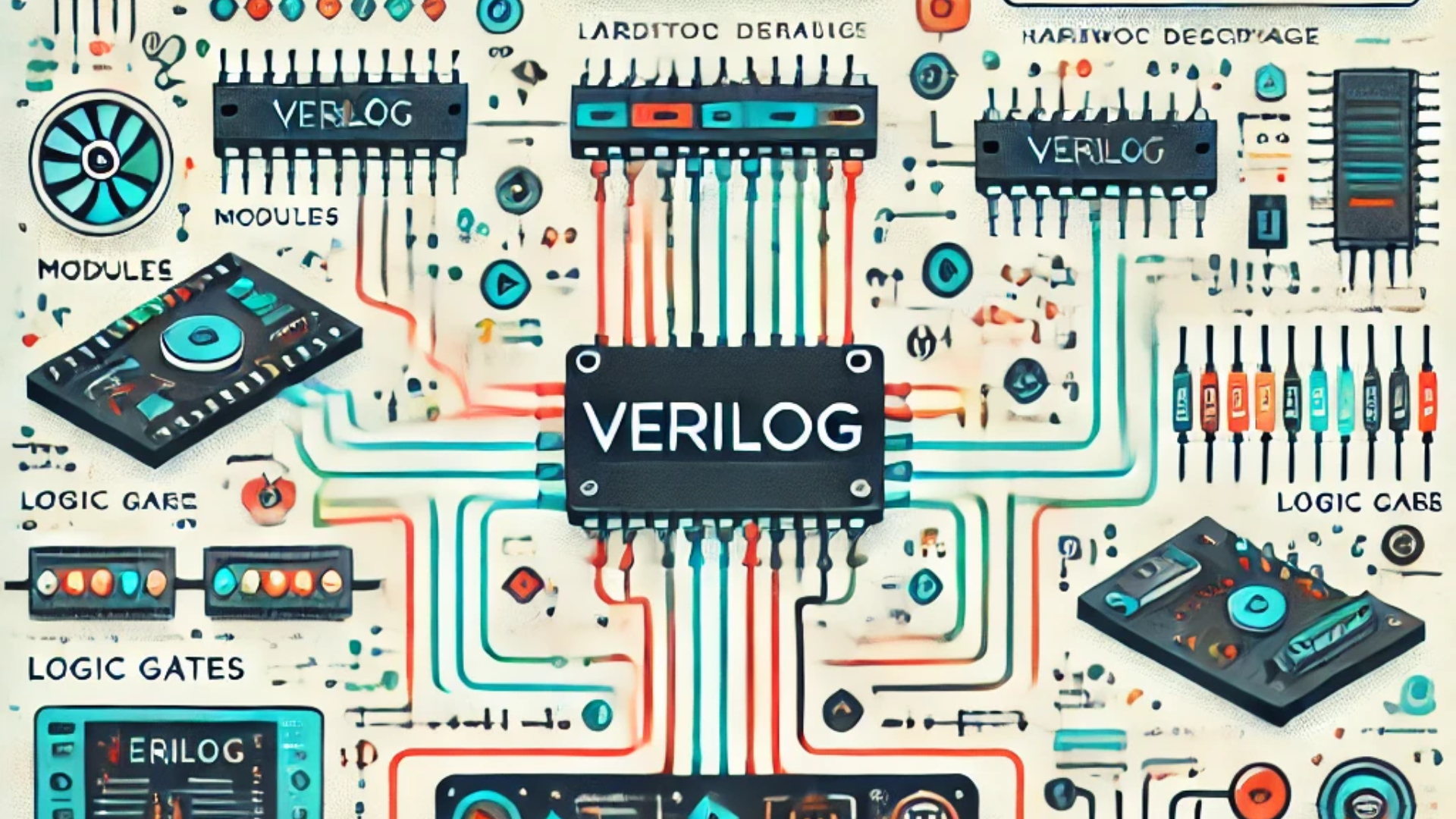
Ever wondered how digital circuits are designed? From the chips in your smartphone to the processors in your computer, digital circuits are everywhere! But how do engineers describe and simulate these circuits before actually building them? That’s where Verilog comes in. Verilog is a hardware description language (HDL) that allows engineers to model and simulate digital systems. In this guide, we’ll break down Verilog in simple terms, so whether you’re a beginner or just curious, you’ll walk away with a solid understanding.
What is Verilog?
Verilog is a language used to describe electronic circuits and systems. Think of it as a way to “code” hardware, similar to how you might write code in Python or C++ to create software. But instead of telling a computer what to do, you’re telling hardware how to behave. Verilog helps engineers design everything from small logic gates to large, complex processors.
Why Use Verilog?
You might wonder, “Why not just build the circuits directly?” Well, designing a circuit on paper or breadboards can be extremely time-consuming and prone to errors. Verilog allows engineers to:
- Simulate designs before they are built. This helps catch errors early.
- Create complex systems using simple building blocks.
- Reuse code for different projects, saving time and effort.
- Automate testing of circuits, ensuring they work as intended.
Basics of Verilog
Before diving into Verilog code, it’s essential to understand a few fundamental concepts.
Modules
In Verilog, everything is built using modules. A module is like a building block. It can represent anything from a simple AND gate to a more complex component like a processor. Here’s an example of a simple module in Verilog:
module and_gate (
input a,
input b,
output y
);
assign y = a & b;
endmodule
In this example, we’ve created a module called and_gate
that takes two inputs (a
and b
) and produces an output (y
). The assign
statement is used to define the logic – in this case, an AND operation.
Data Types
Verilog supports several data types, but the most commonly used ones are:
- wire: Represents a connection between different components.
- reg: Stores a value and is used in sequential logic.
For example:
wire myWire; // Represents a connection
reg myReg; // Stores a value
Operators
Verilog supports many operators, just like in any programming language. Here are a few:
- &: AND operation
- |: OR operation
- ^: XOR operation
- ~: NOT operation
- +: Addition
- –: Subtraction
Always Block
The always
block is crucial in Verilog for describing sequential logic. It tells the hardware to perform certain operations when specific conditions are met. Here’s a simple example:
always @(posedge clk) begin
if (reset)
q <= 0;
else
q <= d;
end
In this code, when the clock (clk
) has a rising edge, the code checks if reset
is active. If it is, the output (q
) is set to 0. Otherwise, q
gets the value of d
.
How to Write a Simple Verilog Code
Let’s walk through writing a basic Verilog code for a 2-to-1 multiplexer (MUX). A MUX is a device that selects one of two inputs to pass through as output based on a control signal.
module mux2to1 (
input wire a, // First input
input wire b, // Second input
input wire sel, // Select signal
output wire y // Output
);
assign y = (sel) ? b : a;
endmodule
In this example:
a
andb
are the inputs.sel
is the control signal.y
is the output.
The assign
statement uses a conditional operator (? :
) to decide whether y
should be a
or b
based on the value of sel
.
Simulating Verilog Code
Writing code is just half the battle; you need to test it! Verilog allows for simulation, where you can test your designs in a virtual environment before committing to physical hardware.
A simple testbench for the MUX might look like this:
module tb_mux2to1;
reg a;
reg b;
reg sel;
wire y;
// Instantiate the MUX
mux2to1 uut (
.a(a),
.b(b),
.sel(sel),
.y(y)
);
initial begin
// Apply test cases
a = 0; b = 1; sel = 0;
#10; // Wait for 10 time units
sel = 1;
#10;
// Check output
$display("Output y = %b", y);
end
endmodule
Here’s what this code does:
- Declares test signals (
a
,b
,sel
) and a wire for the output (y
). - Instantiates the MUX (
mux2to1
) using the signals. - Applies test cases by changing the values of
a
,b
, andsel
over time. - Displays the output using
$display
.
Advantages of Verilog
- Widely Used: Verilog is one of the most popular HDLs, supported by many tools and platforms.
- Simple Syntax: Its syntax is easy to grasp, especially for those familiar with C-like languages.
- Versatile: You can describe everything from simple logic gates to complex state machines.
- Strong Simulation Support: Verilog’s simulation capabilities allow for extensive testing before hardware implementation.
Common Applications
Verilog is used in various applications, including:
- ASIC Design: Application-Specific Integrated Circuits, which are custom chips tailored for a particular application.
- FPGA Design: Field-Programmable Gate Arrays, which are reconfigurable chips used in many modern electronics.
- Embedded Systems: Verilog helps design the core components of embedded systems, like microcontrollers.
- Computer Architecture: Used to design and simulate the processors and memory components in computers.
Tips for Beginners
- Start Simple: Begin with small designs, like basic gates, and gradually move to more complex systems.
- Practice Regularly: The best way to learn Verilog is by writing and simulating your code frequently.
- Use Simulation Tools: Tools like ModelSim or Vivado provide a great way to visualize your designs.
- Read Documentation: Verilog has extensive documentation. Don’t hesitate to refer to it when in doubt.
- Join Online Communities: Forums like Stack Overflow or GitHub can be helpful for troubleshooting and learning from others.
Conclusion
Verilog is a powerful language that plays a critical role in digital circuit design. Whether you’re working on a small project or a large-scale system, understanding Verilog can open up many opportunities in hardware design. Start with the basics, practice regularly, and don’t shy away from diving into more complex designs as you progress.